vscode-http-client


Simple way to do HTTP requests in Visual Studio Code.
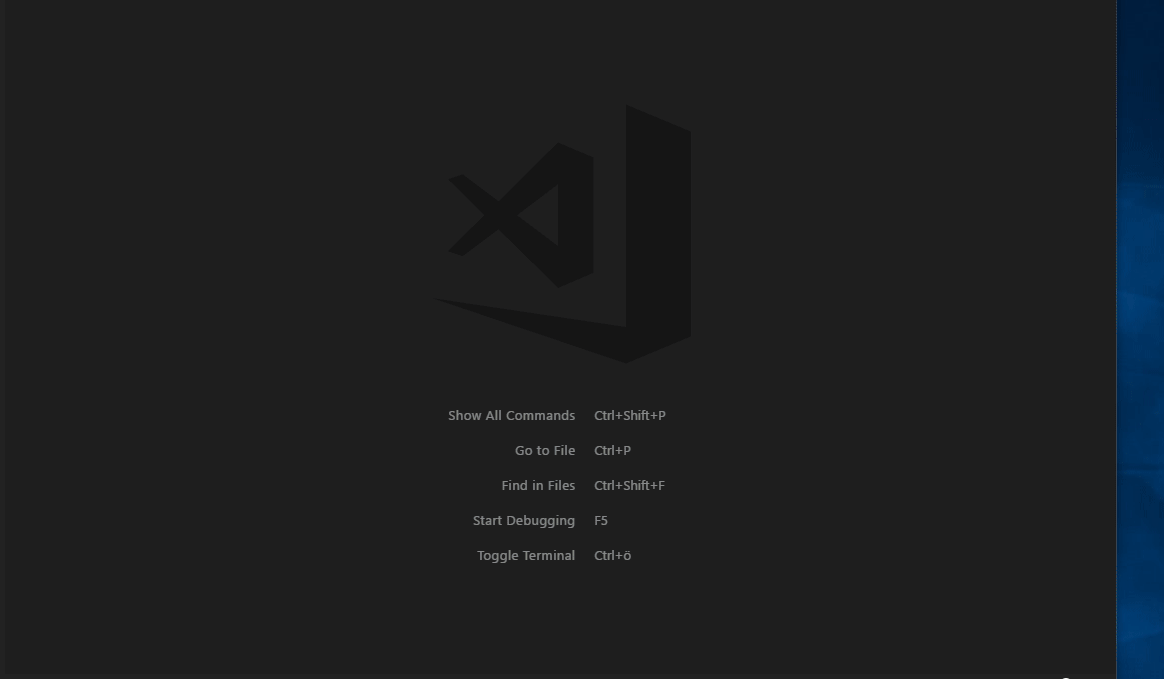
Table of contents
- Install
- How to use
- Enhancements
- HTTP files
- Customizations
- Syntaxes
- Support and contribute
- Related projects
Install [↑]
Launch VS Code Quick Open (Ctrl + P
), paste the following command, and press enter:
ext install vscode-http-client
Or search for things like vscode-http-client
in your editor.
How to use [↑]
Settings [↑]
Open (or create) your settings.json
in your .vscode
subfolder of your workspace or edit the global settings (File >> Preferences >> Settings
).
Add a http.client
section:
{
"http.client": {
}
}
Name |
Description |
open |
An array of one or more paths to .http-request files, which should be opened on startup. |
openNewOnStartup |
(true) , if a new tab with an empty request should be opened on startup. Default: (false) |
rejectUnauthorized |
(true) , to reject unauthorized, self-signed SSL certificates. Default: (false) |
How to execute [↑]
Press F1
and enter one of the following commands:
Name |
Description |
command |
HTTP Client: Change style ... |
Changes the CSS style for request forms. |
extension.http.client.changeStyle |
HTTP Client: Create new script ... |
Opens a new editor with an example script. |
extension.http.client.newRequestScript |
HTTP Client: New HTTP request ... |
Opens a new HTTP request form. |
extension.http.client.newRequest |
HTTP Client: New HTTP request (split view) ... |
Opens a new HTTP request form by splitting the current view. |
extension.http.client.newRequestSplitView |
HTTP Client: Send editor content as HTTP request ... |
Uses the content of a visible editor as body for a HTTP request. |
extension.http.client.newRequestForEditor |
HTTP Client: Send file as HTTP request ... |
Uses a (local) file as body for a HTTP request. |
extension.http.client.newRequestFromFile |
HTTP Client: Show help ... |
Shows a new help tab. |
extension.http.client.showHelp |
There are currently no predefined key bindings for these commands, but you can setup them by your own.
Execute scripts [↑]
For things, like batch operations, you can execute scripts, using the Node.js API, which is provided by VS Code.
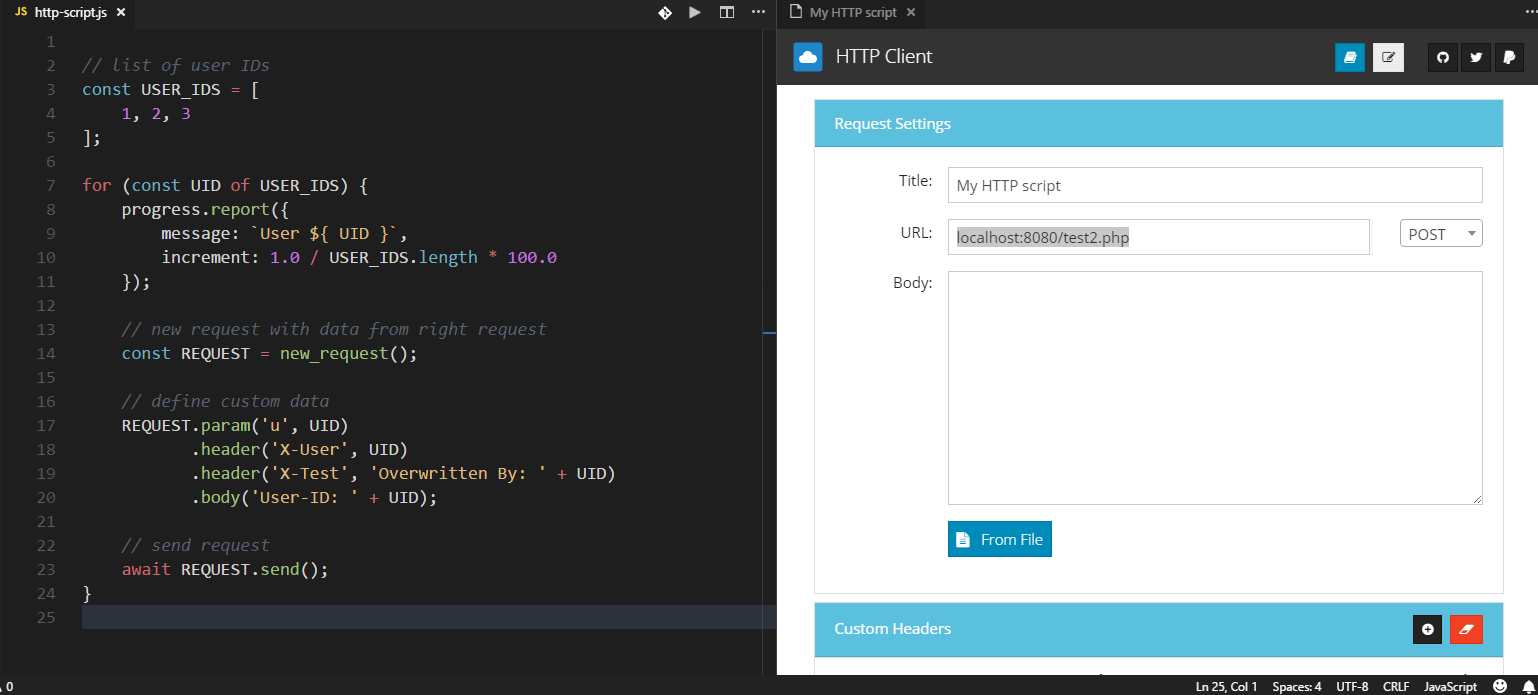
const USERS = [{
id: 5979,
name: 'TM'
}, {
id: 23979,
name: 'MK'
}];
const SESSION_ID = uuid();
const CURRENT_TIME = utc();
// show output channel
output.show();
for (let i = 0; i < USERS.length; i++) {
if (cancel.isCancellationRequested) {
break; // user wants to cancel
}
const U = USERS[i];
try {
output.append(`Sending request for '${ U }' ... `);
const REQUEST = new_request();
// do not show any result
// in the GUI
// this is good, if you do many requests
REQUEST.noResult(true);
REQUEST.param('user', U.id) // set / overwrite an URL / query parameter
.header('X-User-Name', U.name) // set / overwrite a request header
.header('X-Date', CURRENT_TIME) // automatically converts to ISO-8601
.header('X-Session', SESSION_ID)
.body( await $fs.readFile('/path/to/bodies/user_' + U.id + '.json') ); // set / overwrite body
// you can also use one of the upper setters
// as getters
if ('MK' === REQUEST.header('X-User-Name')) {
REQUEST.param('debug', 'true');
}
await REQUEST.send();
output.appendLine(`[OK]`);
} catch (e) {
output.appendLine(`[ERROR: '${ $h.toStringSafe(e) }']`);
}
}
Constants [↑]
cancel [↑]
Provides the underlying CancellationToken object.
const USER_IDS = [1, 2, 3];
for (let i = 0; i < USER_IDS.length; i++) {
if (cancel.isCancellationRequested) {
break; // user wants to cancel
}
// TODO
}
output [↑]
Provides the OutputChannel of the extension.
output.show();
output.append('Hello');
output.appendLine(', TM!');
progress [↑]
Stores the underlying Progress object, provided by withProgress() function.
const USER_IDS = [1, 2, 3];
for (let i = 0; i < USER_IDS.length; i++) {
const UID = USER_IDS[i];
progress.report({
message: `Executing code for user ${ i + 1 } (ID ${ USER_ID }) of ${ USERS.length } ...`,
increment: 1.0 / USERS.length * 100.0
});
// TODO
}
Functions [↑]
alert [↑]
Shows a (warning) popup.
alert('Hello!');
// with custom buttons
switch (await alert('Sure?', 'NO!', 'Yes')) {
case 'NO!':
console.log('User is UN-SURE!');
break;
case 'Yes':
console.log('User is sure.');
break;
}
decode_html [↑]
Decodes the entities in a HTML string.
let encodedStr = '<strong>This is a test!</strong>';
// all entities
decode_html(encodedStr); // '<strong>This is a test!</strong>'
// HTML 4
decode_html(encodedStr, '4');
// HTML 5
decode_html(encodedStr, '5');
// XML
decode_html(encodedStr, 'xml');
encode_html [↑]
Encodes a string to HTML entities.
let strToEncode = '<strong>This is a test!</strong>';
// all entities
encode_html(strToEncode); // '<strong>This is a test!</strong>'
// HTML 4
encode_html(strToEncode, '4');
// HTML 5
encode_html(strToEncode, '5');
// XML
encode_html(strToEncode, 'xml');
from [↑]
Creates a new LINQ style iterator for any iterable object, like arrays, generators or strings. For more information, s. node-enumerable.
const SEQUENCE = from([1, '2', null, 3, 4, undefined, '5']);
const SUB_SEQUENCE = SEQUENCE.where(x => !_.isNil(x)) // 1, '2', 3, 4, '5'
.ofType('string'); // '2', '5'
for (const ITEM of SUB_SEQUENCE) {
// TODO
}
guid [↑]
Generates a new unique ID, using node-uuid.
// v1
const GUID_v1_1 = guid('1');
const GUID_v1_2 = guid('v1');
// v4
const GUID_v4_1 = guid();
const GUID_v4_2 = guid('4');
const GUID_v4_3 = guid('v4');
// v5
const GUID_v5_1 = guid('5');
const GUID_v5_2 = guid('v5');
new_request [↑]
Creates a new instance of a HTTP client, by using the data of the current request form. All important data, like URL, headers or body can be overwritten.
const REQUEST = new new_request();
REQUEST.param('user', 5979) // set / overwrite an URL / query parameter
.header('X-My-Header', 'TM') // set / overwrite a request header
.body( await $fs.readFile('/path/to/body') ); // set / overwrite body
await REQUEST.send();
now [↑]
Returns a new instance of a Moment.js object, by using an optional parameter for the timezone.
const CURRENT_TIME = now();
console.log( CURRENT_TIME.format('YYYY-MM-DD HH:mm:ss') );
const CURRENT_TIME_WITH_TIMEZONE = now('Europe/Berlin');
console.log( CURRENT_TIME_WITH_TIMEZONE.format('DD.MM.YYYY HH:mm') );
open_html [↑]
Opens a new HTML tab.
let htmlCode = `<!doctype html>
<html lang="en">
<head>
<meta charset="utf-8">
<script>
const vscode = acquireVsCodeApi();
</script>
</head>
<body>
<div>Hello, TM!</div>
</body>
</html>`;
// the function returns the underlying webview
// s. https://code.visualstudio.com/docs/extensionAPI/vscode-api#WebviewPanel
html(htmlCode);
// with custom title
html(htmlCode, 'My HTML document');
SECURITY HINT: The new tab is opened with the following settings:
{
"enableCommandUris": true,
"enableFindWidget": true,
"enableScripts": true,
"retainContextWhenHidden": true
}
open_markdown [↑]
Opens a new HTML tab with parsed Markdown content.
let markdownCode = `# Header 1
## Header 2
Lorem ipsum dolor sit amet.
`;
// the function returns the underlying webview
// s. https://code.visualstudio.com/docs/extensionAPI/vscode-api#WebviewPanel
md(markdownCode);
// with custom title
md(markdownCode, 'My Markdown document');
// with custom, optional title and CSS
md(markdownCode, {
css: `
body {
background-color: red;
}
`,
title: 'My Markdown document'
});
The parser uses the following settings:
{
"breaks": true,
"gfm": true,
"mangle": true,
"silent": true,
"tables": true,
"sanitize": true
}
SECURITY HINT: The new tab is opened with the following settings:
{
"enableCommandUris": false,
"enableFindWidget": false,
"enableScripts": true,
"retainContextWhenHidden": true
}
sleep [↑]
Waits a number of seconds before continue.
await sleep(); // 1 second
await sleep(2.5); // 2.5 seconds
uuid [↑]
Alias for guid().
utc [↑]
Returns a new instance of a Moment.js object in UTC.
const UTC_NOW = utc();
console.log( UTC_NOW.format('YYYY-MM-DD HH:mm:ss') );
Modules [↑]
You also can include any module, shipped with VS Code, Node.js, that extension or any external script, which is available on your current system, by using the require()
function.
Enhancements [↑]
Authorization [↑]
Basic Auth [↑]
Instead of setting up a Basic Auth request header by using a Base64 string like
Authorization: Basic bWtsb3ViZXJ0Om15UGFzc3dvcnQ=
you can use the value as simple plain text, separating username and password by :
character:
Authorization: Basic mkloubert:mypassword
Keep in mind: The string right to Basic
will be trimmed!
HTTP files [↑]
Requests can be imported from or to HTTP files.
Those files are encoded in ASCII and their lines are separated by CRLF (\r\n
):
POST https://example.com/users/1 HTTP/1.1
Content-type: application/json; charset=utf8
X-User: 1
{
"id": 1,
"name": "mkloubert"
}
Customizations [↑]
CSS [↑]
You can save a custom CSS file, called custom.css
, inside the extension's folder, which is the sub folder .vscode-http-client
inside the current user's home directory.
Syntaxes [↑]
Syntax highlighting supports all languages, which are part of highlight.js.
Support and contribute [↑]
If you like the extension, you can support the project by sending a donation via PayPal to me.
To contribute, you can open an issue and/or fork this repository.
To work with the code:
- clone this repository
- create and change to a new branch, like
git checkout -b my_new_feature
- run
npm install
from your project folder
- open that project folder in Visual Studio Code
- now you can edit and debug there
- commit your changes to your new branch and sync it with your forked GitHub repo
- make a pull request
node-enumerable [↑]
node-enumerable is a LINQ implementation for JavaScript, which runs in Node.js and browsers.
vscode-helpers [↑]
vscode-helpers is a NPM module, which you can use in your own VSCode extension and contains a lot of helpful classes and functions.