💎 vscode-glsl-canvas

The extension opens a live WebGL preview of GLSL shaders within VSCode by providing a Show glslCanvas
command.
It use glsl-canvas a modified and improved version of glslCanvas javascript library from Book of Shaders and glslEditor made by Patricio Gonzalez Vivo.
Now supporting WebGL2. just add #version 300 es
at the very start of the file.
Now supporting nested includes with relative paths.
Optimized video recording options, with record size and duration. Choose between MediaRecorderApi or CCapture for high quality compression.
Run ⌘ ⇧ P on mac os, ctrl ⇧ P on windows.
Then type Show glslCanvas
command to display a fullscreen preview of your fragment shader.
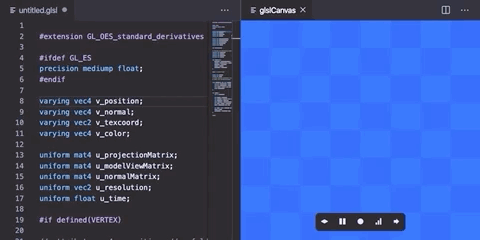
Features
- Both supports WebGL and WebGL2. Automatically create WebGL2 context by adding
#version 300 es
as the first line of file.
- Integrated support of error handling with lines hilight.
- Drawing modes:
flat
, box
, sphere
, torus
and mesh
with default mesh.
- Mesh loader and parser for
.obj
format.
- Vertex in fragment with
VERTEX
macro.
- Multiple buffers with
BUFFER
macro.
- Play / pause functionality.
- Recording and exporting to
.webm
video with resolution and duration settings, using MediaRecorder
or CCapture
for high quality video.
- Activable stats.js performance monitor.
- Custom uniforms.
- Activable gui for changing custom uniforms at runtime.
- Export to html page with live reload.
- Glsl code formatter standard and compact mode.
- Glsl Snippets.
Attributes
The attributes provided are a_position
, a_normal
, a_texcoord
, a_color
.
Type |
Property |
vec4 |
a_position |
vec4 |
a_normal |
vec2 |
a_texcoord |
vec4 |
a_color |
The uniforms provided are u_resolution
, u_time
, u_mouse
, u_camera
and u_trails[10]
.
Type |
Property |
vec2 |
u_resolution |
float |
u_time |
vec2 |
u_mouse |
vec3 |
u_camera |
vec2[10] |
u_trails[10] |
WebGL Extensions
You can include any supported WebGL extension through the glsl-canvas.extensions
array option modifying the workspace's settings.json
file.
{
"glsl-canvas.extensions": [
"EXT_shader_texture_lod"
]
}
You can then enable the extension in the shader
#extension GL_EXT_shader_texture_lod : enable
vec3 color = texture2DLodEXT(u_texture, st, 0.0).rgb;
WebGL2
For WebGL2 context creation just add #version 300 es
at the very start of the file.
no other characters allowed before macro!
#version 300 es
precision mediump float;
IO Buffers
You can use shader buffers by requiring definition with #ifdef
or defined
directly in .glsl
code.
Just ask for BUFFER_
N definition and a u_buffer
N uniform will be created for you:
uniform sampler2D u_buffer0;
#ifdef BUFFER_0
void main() {
vec4 color = texture2D(u_buffer0, uv, 0.0);
...
gl_FragColor = color;
}
#else
void main() {
vec4 color = texture2D(u_buffer0, uv, 0.0);
...
gl_FragColor = color;
}
#endif
Playground
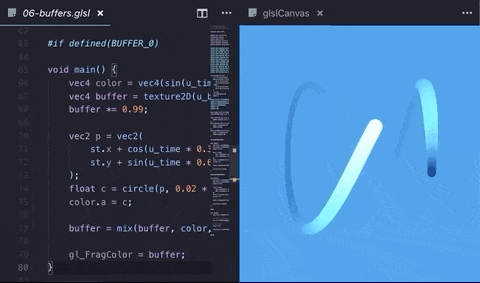
Textures
You can define the texture channels (u_texture_0
, u_texture_1
, ...) by modifying the workspace's settings.json
file.
{
"glsl-canvas.textures": {
"0": "./texture.png",
"1": "https://rawgit.com/actarian/plausible-brdf-shader/master/textures/noise/cloud-1.png",
"2": "https://rawgit.com/actarian/plausible-brdf-shader/master/textures/noise/cloud-2.jpg",
}
}
As of today VSCode do not support video element or audio element. You can use video texture with the Export to html feature.
u_camera
u_camera
is a vec3 array with coordinates for an orbital camera positioned at world zero, useful for raymarching.
Playground
u_trails[10]
u_trails[10]
is a vec2 array with stored inertia mouse positions for mouse trailing effects.
Playground
You can also define custom uniforms by modifying the workspace's settings.json
file.
{
"glsl-canvas.uniforms": {
"u_strength": 1.0
}
}
Types supported are float
, vec2
, vec3
and vec4
. Vectors structures are converted from arrays of floats. Range values goes from 0.0
to 1.0
.
Type |
Property |
float |
"u_float": 1.0, |
vec2 |
"u_vec2": [1.0, 1.0], |
vec3 |
"u_vec3": [1.0, 1.0, 1.0], |
vec4 |
"u_vec4": [1.0, 1.0, 1.0, 1.0], |
By clicking the option button you can view and modify at runtime uniforms via the dat.gui interface.
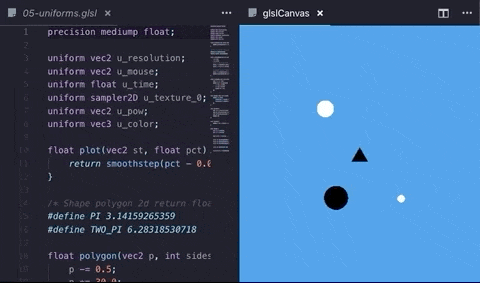
Export to html
You can export your shader, assets and uniforms to an html page with livereload for testing in browser.
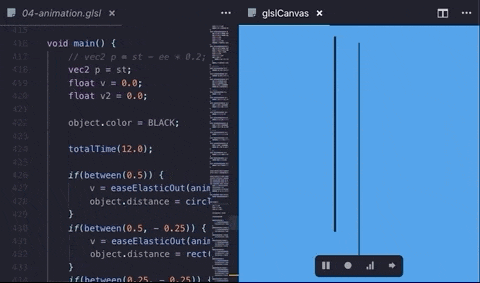
Color Picker
Waiting for a more customizable code inset feature, vec3
and vec4
arrays can be modified with the integrated color picker.
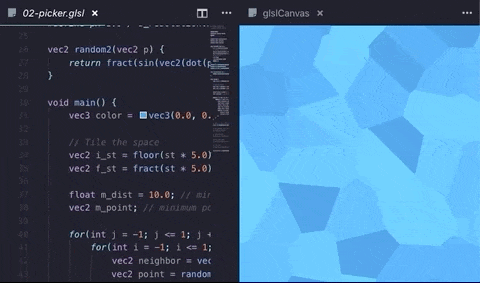
Including dependent files with #include
You can now include other GLSL code using a traditional #include "file.glsl"
macro.
// example
#include "common/uniforms.glsl"
#include "common/functions.glsl"
void main(){
Antialias
Enables or disables antialias in the WebGL context.
{
"glsl-canvas.antialias": false
}
Change detection
You can set the timeout change detection option by modifying the workspace's settings.json
file.
{
"glsl-canvas.timeout": 0
}
Refresh on save
Enables or disables refreshing the glslCanvas when saving the document.
{
"glsl-canvas.refreshOnSave": true
}
Refresh on change
Enables or disables refreshing the glslCanvas when changing the document.
{
"glsl-canvas.refreshOnChange": true
}
Enables or disables glsl code formatter.
{
"glsl-canvas.useFormatter": true
}
Enables or disables glsl code formatter compact mode.
{
"glsl-canvas.useCompactFormatter": false
}
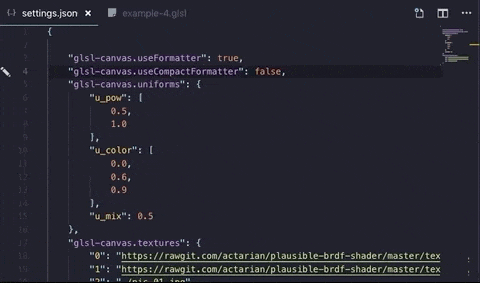
Install on export
Enables or disables installing the Npm packages on export.
{
"glsl-canvas.installOnExport": true
}
Record duration
Specify automatic recording duration in seconds. Set to 0
to disable this feature.
{
"glsl-canvas.recordDuration": 10
}
Record width
Specify canvas recording width in pixels. Default value is 1024
.
{
"glsl-canvas.recordWidth": 1024
}
Record height
Specify canvas recording height in pixels. Default value is 1024
.
{
"glsl-canvas.recordHeight": 1024
}
Fragment shader
An example of fragment shader. You can copy paste this code in an empty .glsl
file:
precision mediump float;
uniform vec2 u_resolution;
uniform vec2 u_mouse;
uniform float u_time;
void main() {
vec2 st = gl_FragCoord.xy / u_resolution.xy;
st.x *= u_resolution.x / u_resolution.y;
vec3 color = vec3(0.0);
color = vec3(st.x, st.y, abs(sin(u_time)));
gl_FragColor = vec4(color, 1.0);
}
Glsl snippets
Snippet |
Purpose |
glsl.animation |
Staggered animations |
glsl.modifiers.blend |
Blend functions |
glsl.modifiers.boolean |
Boolean functions |
glsl.colors |
Colors palette |
glsl.coords |
Pixel units utility functions |
glsl.drawing |
Signed distance drawing methods |
glsl.ease.back.in |
Ease equation back in |
glsl.ease.bounce.in |
Ease equation bounce in |
glsl.ease.circular.in |
Ease equation circular in |
glsl.ease.cubic.in |
Ease equation cubic in |
glsl.ease.elastic.in |
Ease equation elastic in |
glsl.ease.expo.in |
Ease equation expo in |
glsl.ease.quad.in |
Ease equation quad in |
glsl.ease.quart.in |
Ease equation quart in |
glsl.ease.quint.in |
Ease equation quint in |
glsl.ease.sine.in |
Ease equation sine in |
glsl.main.new |
Main function, uniforms & utils |
glsl.core.object |
Object struct with distance and color |
glsl.shapes.2d.arc |
Shape 2D arc |
glsl.shapes.2d.circle |
Shape 2D circle |
glsl.shapes.2d.grid |
Shape 2D grid |
glsl.shapes.2d.hex |
Shape 2D hexagon |
glsl.shapes.2d.line |
Shape 2D line |
glsl.shapes.2d.pie |
Shape 2D pie |
glsl.shapes.2d.plot |
Shape 2D plot |
glsl.shapes.2d.poly |
Shape 2D poly |
glsl.shapes.2d.rect |
Shape 2D rect |
glsl.shapes.2d.roundrect |
Shape 2D roundrect |
glsl.shapes.2d.segment |
Shape 2D segment |
glsl.shapes.2d.spiral |
Shape 2D spiral |
glsl.shapes.2d.star |
Shape 2D star |
glsl.modifiers.tile |
Tiling function |
glsl.units |
Pixel unit conversion function |
Snippets library documentation and playgrounds here.
Requirements
- A graphics card supporting WebGL.
Todo
Contributing
Pull requests are welcome and please submit bugs 🐞
Thank you for taking the time to provide feedback and review. This feedback is appreciated and very helpful 🌈


Release Notes
Changelog here.