Skaberen code generator extension for Visual Studio Code
Skaberen code generator is an extension of Visual Studio Code that allows us to generate a base code with a graphical user interface.
Inspired by Angular files
Why this extension?
This extension will save you time:
- Spring boot: Generates a complete REST API, creating entities, services, repositories and controllers.
- NgRx: Generates actions, effects and reducers simply by indicating the name of the model.
- Spring Security (Beta): Generates an initial code to authenticate with JWT token.
Requirements
Result
[DEMO 1]
https://github.com/SkaberenWorm/demo1-skaberen-code-generator (Use ResultadoProc class)
[DEMO 2] https://github.com/SkaberenWorm/demo2-skaberen-code-generator - recommended
Result - Use ResultadoProc class (DEMO 1)
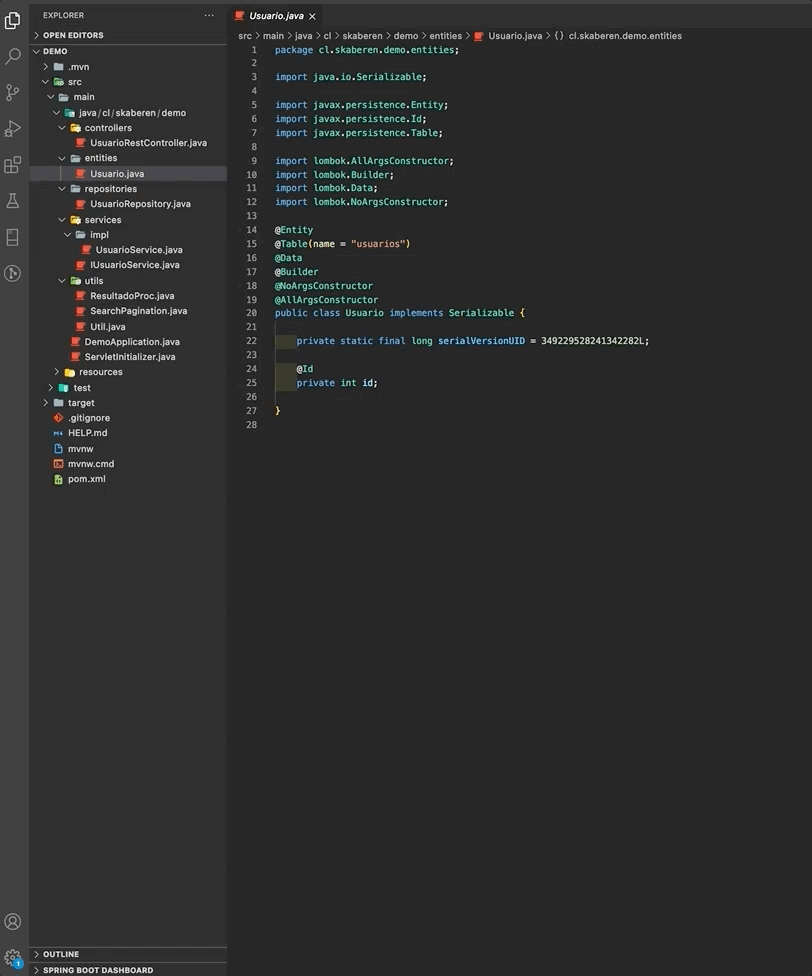
Getting started
API REST with Spring boot
Right-click on the main package in the directory browser, then select "Skaberen Java: Generate base code"
NOTE: The path of the selected directory will be taken as the main package
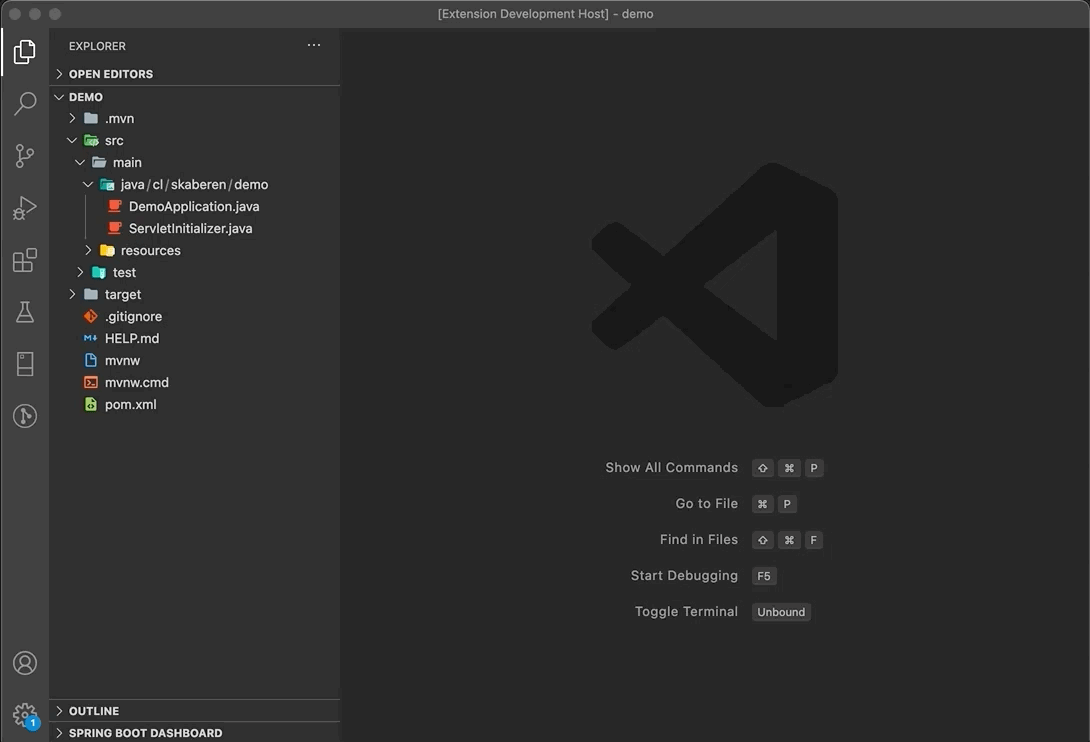
How to enable the option to use the ResultadoProc class?
You must add this line in your vscode configuration file (settings.json)
{
"skaberen.useResuldadoProc": true,
...
}
More information: https://code.visualstudio.com/docs/getstarted/settings#_settingsjson
What's this?
Here you can select how you want your code to be written.
If you select "NO usar clase ResultadoProc", Your code will look like this.
// Controller
@GetMapping("/{id}")
public ResponseEntity<Usuario> findById(@PathVariable("id") int usuarioId)
throws ErrorProcessingException, EntityNotFoundException {
Usuario salida = usuarioService.findById(usuarioId);
return new ResponseEntity<Usuario>(salida, HttpStatus.OK);
}
// Service
@Override
public Usuario findById(final int usuarioId) throws ErrorProcessingException, EntityNotFoundException {
try {
return this.usuarioRepository.findById(usuarioId).orElseThrow(() -> new EntityNotFoundException());
} catch (final EntityNotFoundException e) {
throw new EntityNotFoundException();
} catch (final Exception e) {
throw new ErrorProcessingException();
}
}
The response will look like this
// Status: 200 OK
{
"rut": "12345678-0",
"nombre": "Ismael",
"apellido": "Cuevas",
"email": "ismael.c.26a@gmail.com"
}
// Status: 404 Not found
{
"status": 404,
"timestamp": "2021-04-18T16:37:08.819-04:00",
"error": "NOT_FOUND",
"message": "El registro solicitado no existe",
"path": "uri=/api/usuario/12345678-9"
}
but if you select "Usar clase ResultadoProc", Your code will look like this.
// Controller
@GetMapping("/{id}")
public ResponseEntity<ResultadoProc<Usuario>> findById(@PathVariable("id") int usuarioId) {
ResultadoProc<Usuario> salida = usuarioService.findById(usuarioId);
return new ResponseEntity<ResultadoProc<Usuario>>(salida, HttpStatus.OK);
}
// Service
@Override
public ResultadoProc<Usuario> findById(final int usuarioId) {
final ResultadoProc.Builder<Usuario> salida = new ResultadoProc.Builder<Usuario>();
try {
final Usuario usuario = this.usuarioRepository.findById(usuarioId).orElse(null);
if (usuario == null) {
salida.fallo("Usuario no encontrado");
}
salida.exitoso(usuario);
} catch (final Exception e) {
Util.printError("findById(" + usuarioId + ")", e);
salida.fallo("Se produjo un error inesperado al intentar obtener usuario");
}
return salida.build();
}
The response will look like this
// Status: 200 OK
{
"error": "false",
"mensaje": "",
"resultado": {
"rut": "12345678-0",
"nombre": "Ismael",
"apellido": "Cuevas",
"email": "ismael.c.26a@gmail.com"
}
}
// Status: 200 OK
// "error": "true" -> Error true
{
"error": "true",
"mensaje": "Usuario no encontrado",
"resultado": null
}