Monokai X
Install Jetbrains Mono
Font
Some monospace fonts from Google Fonts. For instance, to make JetBrains Mono font family available for Visual Studio Code editor, you have to download and install into your system by follow below instructions:
Install Additional Extensions
Python users may be pleased to test the flavor of the following extensions:
- Code Runner by Jun Han
- CodeSnap by adpyke
- Error Lens by Alexander
- Excel Viewer by GrapeCity
- FontSize Viewer by Foss & Haas
- indent-rainbow by oderwat
- IntelliCode Completions by Microsoft
- Jupyter by Microsoft
- Jupyter Cell Tags by Microsoft
- Jupyter Keymap by Microsoft
- Jupyter Notebook Rederers by Microsoft
- Jupyter Slide Show by Microsoft
- Material Icon Theme by Philipp Kief
- Pylance by Microsoft
- Python by Microsoft
- Rainbow CSV by cechatroner
- vscode-pdf by romoki207
Install Additional Kernels
To use the Jupyter Notebook
and Code Formatter
, Python users have to install ipykernel
and autopep8
. To achieve this, launch Terminal
or Command Prompt
and run the following commands.
pip install autopep8
pip install ipykernel
- Shortcut key:
Ctrl+Shift+P
to open Command Pallete
- Input
>Preferences: open user settings (JSON)
- Add the following customizations to
settings.json
:
{
"git.autofetch": true,
"window.commandCenter": true,
"files.trimFinalNewlines": true,
"files.trimTrailingWhitespace": true,
"files.autoSave": "afterDelay",
"workbench.colorTheme": "Monokai X",
"workbench.iconTheme": "material-icon-theme",
"fontshortcuts.step": 2,
"fontshortcuts.defaultFontSize": 18,
"fontshortcuts.defaultTerminalFontSize": 18,
"editor.fontSize": 18,
"editor.lineHeight": 30,
"editor.fontFamily": "JetBrains Mono",
"editor.fontLigatures": false,
"editor.fontWeight": 400,
"editor.letterSpacing": 1,
"editor.tabSize": 4,
"editor.mouseWheelZoom": false,
"editor.glyphMargin": false,
"editor.lineNumbers": "on",
"editor.minimap.enabled": false,
"editor.formatOnType": true,
"editor.formatOnSave": true,
"editor.formatOnPaste": true,
"editor.hover.enabled": true,
"editor.tabCompletion": "on",
"editor.renderLineHighlight": "gutter",
"editor.parameterHints.enabled": false,
"editor.accessibilitySupport": "on",
"editor.cursorBlinking": "expand",
"editor.cursorWidth": 4,
"jupyter.interactiveWindow.creationMode": "perFile",
"notebook.output.textLineLimit": 120,
"notebook.output.lineHeight": 0,
"notebook.markup.fontSize": 16,
"notebook.output.fontSize": 16,
"notebook.output.scrolling": true,
"notebook.formatOnSave.enabled": true,
"notebook.lineNumbers": "on",
"notebook.insertToolbarLocation": "both",
"notebook.cellToolbarLocation": {
"default": "hidden"
},
"[python]": {
"editor.defaultFormatter": "ms-python.python"
},
"python.formatting.provider": "autopep8",
"python.formatting.autopep8Args": [
"--experimental",
"--max-line-length=120"
],
"indentRainbow.indicatorStyle": "classic",
"indentRainbow.ignoreErrorLanguages": [
"python"
],
"indentRainbow.colors": [
"rgba(255,255,64,0.2)",
"rgba(127,255,127,0.2)",
"rgba(255,127,255,0.2)",
"rgba(79,236,236,0.2)"
],
"codesnap.target": "window",
"codesnap.roundedCorners": true,
"codesnap.showWindowTitle": true,
"codesnap.realLineNumbers": true,
"codesnap.containerPadding": "1em",
"code-runner.clearPreviousOutput": true,
"[code-runner-output]": {
"editor.fontSize": 16,
},
"audioCues.volume": 50,
"audioCues.notebookCellCompleted": "off",
"audioCues.notebookCellFailed": "off",
"audioCues.diffLineDeleted": "off",
"audioCues.diffLineInserted": "off",
"audioCues.diffLineModified": "off",
"audioCues.lineHasBreakpoint": "off",
"audioCues.lineHasError": "off",
"audioCues.lineHasFoldedArea": "off",
"audioCues.lineHasInlineSuggestion": "off",
"audioCues.noInlayHints": "off",
"audioCues.onDebugBreak": "off",
"audioCues.taskCompleted": "off",
"audioCues.taskFailed": "off",
"audioCues.terminalCommandFailed": "off",
"audioCues.terminalQuickFix": "off",
}
Screenshot
After following above instruction, your Visual Studion Code should look like:

Sample Code
This is RungeKutta2.py
sample code.
import numpy as np
import pandas as pd
from collections.abc import Callable
from typing import Literal
def RungeKutta2(f: Callable[[np.float64, np.float64], np.float64],
t_span: np.ndarray,
y_init: np.float64,
n: int,
method: Literal["Midpoint", "Heun", "Ralston"]
) -> pd.DataFrame:
if method == "Midpoint":
c = np.array(object=[0, 1/2], dtype=np.float64)
a = np.array(object=[[0, 0],
[1/2, 0]],
dtype=np.float64)
b = np.array(object=[0, 1], dtype=np.float64)
elif method == "Heun":
c = np.array(object=[0, 1], dtype=np.float64)
a = np.array(object=[[0, 0],
[1, 0]],
dtype=np.float64)
b = np.array(object=[1/2, 1/2], dtype=np.float64)
elif method == "Ralston":
c = np.array(object=[0, 2/3], dtype=np.float64)
a = np.array(object=[[0, 0],
[2/3, 0]],
dtype=np.float64)
b = np.array(object=[1/4, 3/4], dtype=np.float64)
h = (t_span[1]-t_span[0]) / n
t = np.linspace(start=t_span[0], stop=t_span[1], num=n+1, dtype=np.float64)
y = np.full_like(a=t, fill_value=np.nan, dtype=np.float64)
y[0] = y_init
for i in range(0, n, 1):
k0 = f(t[i], y[i])
k1 = f(t[i]+c[1]*h, y[i]+(a[1, 0]*k0)*h)
y[i+1] = y[i] + h*(b[0]*k0 + b[1]*k1)
df = pd.DataFrame(data={"t": t, "y": y}, dtype=np.float64)
return df
if __name__ == '__main__':
def f(t: np.float64, y: np.float64) -> np.float64:
return 2*(1-t*y) / (t**2+1)
t_span = np.array(object=[0, 1], dtype=np.float64)
y_init = 1.0
n = 10
def y(t: np.float64) -> np.float64:
return (2*t+1) / (t**2+1)
t = np.linspace(start=t_span[0],
stop=t_span[1],
num=n+1,
dtype=np.float64)
df = pd.DataFrame(data={"t": t}, dtype=np.float64)
df["y_exact"] = df["t"].apply(func=y)
methods = ["Midpoint", "Heun", "Ralston"]
for method in methods:
df_method = RungeKutta2(f=f,
t_span=t_span,
y_init=y_init,
n=n,
method=method)
df_method.rename(columns={"y": f"y_{method}"},
inplace=True)
df_method[f"e_{method}"] = abs(df["y_exact"]-df_method[f"y_{method}"])
df = pd.concat(objs=(df, df_method[[f"y_{method}", f"e_{method}"]]),
axis="columns")
pd.options.display.float_format = "{:.10f}".format
print(df)
df.style.format(precision=10).to_latex("RungeKutta2.tex")
YouTube Video
Click the screenshot to watch the video.
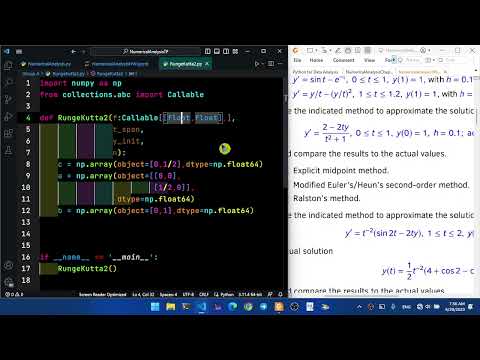