json to dart (JtoD)
Welcome to json to dart (JtoD), yet another code generator for data-classes.
Motivation
- convert json to dart data model
- override toString, operator ==, hashCode
- implement a copyWith method to clone the object
- handling de/serialization
- define a constructor + the properties
- Im Mutable and Mutable data types
Usage
- create data model dart file
- paste json
- run the command "JSON to Dart" or alt+d (keyboard shortcut)
- Enter a class name by default is AutoGeneratedCode
Command to execute is
(win or cmd + shift + p) then enter "JSON to dart" Or alt+d
NOTE: key board shortcut is "alt + d"
Examples
Example 1
{
"name":"praveenD",
"age":"22",
"isAdmin":true,
"order_ids":[1,2,3,4,9,7]
}
Result (user.dart)
class User {
final String? name;
final String? age;
final bool? isAdmin;
final List<int>? orderIds;
const User({this.name, this.age, this.isAdmin, this.orderIds});
User copyWith(
{String? name, String? age, bool? isAdmin, List<int>? orderIds}) {
return User(
name: name ?? this.name,
age: age ?? this.age,
isAdmin: isAdmin ?? this.isAdmin,
orderIds: orderIds ?? this.orderIds);
}
Map<String, Object?> toJson() {
return {'name': name, 'age': age, 'isAdmin': isAdmin, 'orderIds': orderIds};
}
static User fromJson(Map<String, Object?> json) {
return User(
name: json['name'] == null ? null : json['name'] as String,
age: json['age'] == null ? null : json['age'] as String,
isAdmin: json['isAdmin'] == null ? null : json['isAdmin'] as bool,
orderIds:
json['orderIds'] == null ? null : json['orderIds'] as List<int>);
}
@override
String toString() {
return '''User(
name:$name,
age:$age,
isAdmin:$isAdmin,
orderIds:$orderIds
) ''';
}
@override
bool operator ==(Object other) {
return other is User &&
other.runtimeType == runtimeType &&
other.name == name &&
other.age == age &&
other.isAdmin == isAdmin &&
other.orderIds == orderIds;
}
@override
int get hashCode {
return Object.hash(runtimeType, name, age, isAdmin, orderIds);
}
}
Example 2
{
"name":"praveen",
"age":22,
"isAdmin":true,
"order":{
"order_id":233,
"product_name":"productNAME",
"product_price":233.4
}
}
Result Page (product.dart)
class Product {
final String? name;
final int? age;
final bool? isAdmin;
final Order? order;
const Product({this.name, this.age, this.isAdmin, this.order});
Product copyWith({String? name, int? age, bool? isAdmin, Order? order}) {
return Product(
name: name ?? this.name,
age: age ?? this.age,
isAdmin: isAdmin ?? this.isAdmin,
order: order ?? this.order);
}
Map<String, Object?> toJson() {
return {
'name': name,
'age': age,
'isAdmin': isAdmin,
'order': order?.toJson()
};
}
static Product fromJson(Map<String, Object?> json) {
return Product(
name: json['name'] == null ? null : json['name'] as String,
age: json['age'] == null ? null : json['age'] as int,
isAdmin: json['isAdmin'] == null ? null : json['isAdmin'] as bool,
order: json['order'] == null
? null
: Order.fromJson(json['order'] as Map<String, Object?>));
}
@override
String toString() {
return '''Product(
name:$name,
age:$age,
isAdmin:$isAdmin,
order:${order.toString()}
) ''';
}
@override
bool operator ==(Object other) {
return other is Product &&
other.runtimeType == runtimeType &&
other.name == name &&
other.age == age &&
other.isAdmin == isAdmin &&
other.order == order;
}
@override
int get hashCode {
return Object.hash(runtimeType, name, age, isAdmin, order);
}
}
class Order {
final int? orderId;
final String? productName;
final double? productPrice;
const Order({this.orderId, this.productName, this.productPrice});
Order copyWith({int? orderId, String? productName, double? productPrice}) {
return Order(
orderId: orderId ?? this.orderId,
productName: productName ?? this.productName,
productPrice: productPrice ?? this.productPrice);
}
Map<String, Object?> toJson() {
return {
'orderId': orderId,
'productName': productName,
'productPrice': productPrice
};
}
static Order fromJson(Map<String, Object?> json) {
return Order(
orderId: json['orderId'] == null ? null : json['orderId'] as int,
productName:
json['productName'] == null ? null : json['productName'] as String,
productPrice: json['productPrice'] == null
? null
: json['productPrice'] as double);
}
@override
String toString() {
return '''Order(
orderId:$orderId,
productName:$productName,
productPrice:$productPrice
) ''';
}
@override
bool operator ==(Object other) {
return other is Order &&
other.runtimeType == runtimeType &&
other.orderId == orderId &&
other.productName == productName &&
other.productPrice == productPrice;
}
@override
int get hashCode {
return Object.hash(runtimeType, orderId, productName, productPrice);
}
}
Demo
example 1
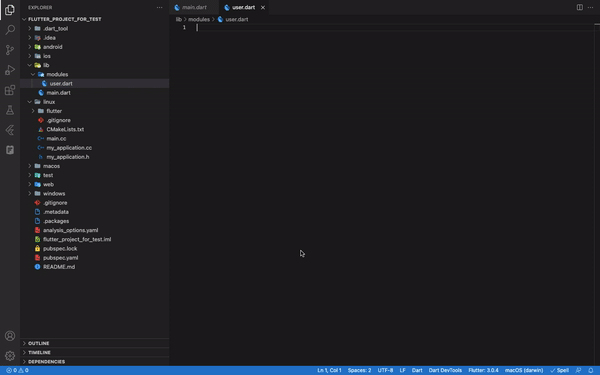
example 2
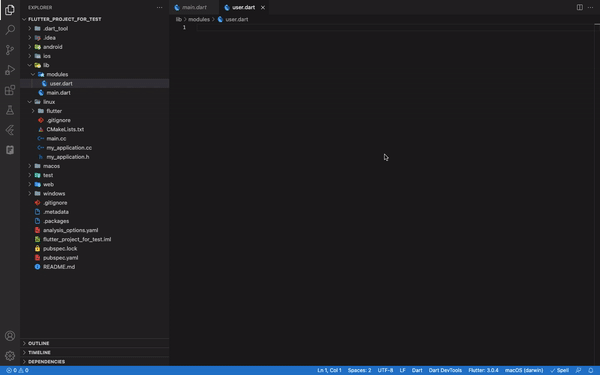