The tasks currently provide:
- Creating and updating comments
- Assigning tags
- Removing tags
- Checking if tag is assigned
- Creating/Updating custom statuses
- Updating pull request description
❗ This extension requires the user account <project name> Build Service (<org name>)
to be granted the permission Contribute to pull requests
Please report any feedback/issue here
📦 Tasks
- PullRequestComments
- PullRequestTags
- PullRequestStatus
- PullRequestDescription
Add comments to a pull request
YAML Snippet
- task: PullRequestComments@0
inputs:
action: create #Action to perform
skipIfCommentExists: true #If the comment exists on the pull request, do not post it again
commentId: #Is used to uniqely identify the comment when using the same task multiple times in the same pipeline
status: Active #Post the comment with a given status
content: #The content of the comment. For Markdown syntax, see [Syntax guidance for basic Markdown usage](http://go.microsoft.com/fwlink/?LinkId=823918)
useDefined: false #If set, overrides the value from `System.PullRequest.PullRequestId`
pullRequestId: $(System.PullRequest.PullRequestId) #If no id is given, the value from `System.PullRequest.PullRequestId` is taken. If a value is given, this overrides the value from `System.PullRequest.PullRequestId`
type: Text #The type of comment. `Text` represents a regular user comment while `System` indicates a system message
Arguments
Argument |
Description |
action Action |
(Optional) Action to perform Options: create , createOrUpdate , update Default value: create |
skipIfCommentExists Do not post comment if it exists |
(Optional) If the comment exists on the pull request, do not post it again Default value: true |
commentId Comment Id |
(Optional) Is used to uniqely identify the comment when using the same task multiple times in the same pipeline
|
status Comment Status |
(Optional) Post the comment with a given status Options: Active , Fixed , WontFix , Closed , Pending Default value: Active |
content Comment Content |
(Required) The content of the comment. For Markdown syntax, see Syntax guidance for basic Markdown usage
|
useDefined Use defined id |
(Optional) If set, overrides the value from System.PullRequest.PullRequestId
|
pullRequestId Pull Request Id |
(Optional) If no id is given, the value from System.PullRequest.PullRequestId is taken. If a value is given, this overrides the value from System.PullRequest.PullRequestId Default value: $(System.PullRequest.PullRequestId) |
type Comment Type (Deprecated) |
(Optional) The type of comment. Text represents a regular user comment while System indicates a system message Options: Text , System Default value: Text |
Examples
Given the following pipeline configuration
steps:
- task: PullRequestComments@0
displayName: 'Post a pull request comment'
inputs:
content: 'This is a comment posted from pipeline $(Build.Repository.Name)'
status: 'Pending'
action: 'create'
after a run against a pull request it will post the comment:
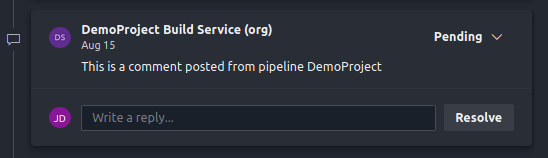
Given the following pipeline configuration
steps:
- task: PullRequestComments@0
inputs:
status: 'Closed'
action: 'create'
content: |
#Title
This is a pull request from `$(Build.DefinitionName)`
| Id | Name |
| --- | ------- |
| 1 | Content |
after a run against a pull request it will post the comment:

When posting multiple comments, ensure commentId
is set to a unique value for each task.
steps:
- task: PullRequestComments@0
displayName: 'Post regular comment'
inputs:
action: 'createOrUpdate'
status: 'Closed'
commentId: 'regular_comment'
content: |
#Title
This is a pull request from `$(Build.DefinitionName)`
| Id | Name |
| --- | ------- |
| 1 | Content |
- task: PullRequestComments@0
displayName: 'Post markdown comment'
inputs:
action: 'createOrUpdate'
status: 'Pending'
commentId: 'markdown_comment'
content: 'This is a comment posted from pipeline $(Build.Repository.Name)'
Manage labels for a pull request. Gives the ability to manage pull request tags, or check if a tag is assigned.
YAML Snippet
- task: PullRequestTags@0
inputs:
action: assign
tag: #Tag to perform action on.
outputVariable: PullRequest.TagCheckResult #The name of the output variable containing the check result
isOutput: false #If set, `outputVariable` is set as output and accessible from other jobs
useDefined: false #If set, overrides the value from `System.PullRequest.PullRequestId`
pullRequestId: $(System.PullRequest.PullRequestId) #If no id is given, the value from `System.PullRequest.PullRequestId` is taken. If a value is given, this overrides the value from `System.PullRequest.PullRequestId`
Arguments
Argument |
Description |
action Action |
(Optional) Options: assign , check , delete Default value: assign |
tag Tag |
(Required) Tag to perform action on.
|
outputVariable Output Variable |
(Required) The name of the output variable containing the check result Default value: PullRequest.TagCheckResult |
isOutput Is output |
(Optional) If set, outputVariable is set as output and accessible from other jobs
|
useDefined Use defined id |
(Optional) If set, overrides the value from System.PullRequest.PullRequestId
|
pullRequestId Pull Request Id |
(Optional) If no id is given, the value from System.PullRequest.PullRequestId is taken. If a value is given, this overrides the value from System.PullRequest.PullRequestId Default value: $(System.PullRequest.PullRequestId) |
Examples
Assign tag to pull request
Given the following pipeline configuration
steps:
- task: PullRequestTags@0
inputs:
tag: 'my-tag'
it will assign the tag my-tag
to the pull request
Remove tag from pull request
Given the following pipeline configuration
steps:
- task: PullRequestTags@0
inputs:
action: 'delete'
tag: 'my-tag'
it will remove the tag my-tag
from the pull request
Check if tag is assigned to pull request
Given the following pipeline configuration
steps:
- task: PullRequestTags@0
name: 'check_tag'
inputs:
action: 'check'
tag: 'my-tag'
outputVariable: 'PullRequest.TagCheckResult'
isOutput: true
it will check if the tag my-tag
is assiged to the active pull request and output the result (true
/ false
) to the variable PullRequest.TagCheckResult
Pull Request Status
PullRequestStatus allows you to create custom statuses for your pull request. See the status policy docs for more infomation.
YAML Snippet
- task: PullRequestStatus@0
inputs:
action: Create
name: my-custom-gate #Name of the status. Full status will be `pull-request-utils/<name>`
description: #Status description. Normally describes the current state of the status.
state: notSet
useDefined: false #If set, overrides the value from `System.PullRequest.PullRequestId`
pullRequestId: $(System.PullRequest.PullRequestId) #If no id is given, the value from `System.PullRequest.PullRequestId` is taken. If a value is given, this overrides the value from `System.PullRequest.PullRequestId`
whenState: #Only update the state of the status when the existing state is one of these
Arguments
Argument |
Description |
action Action |
(Required) Options: Create , Update , Delete Default value: Create |
name Name |
(Required) Name of the status. Full status will be pull-request-utils/<name> Default value: my-custom-gate |
description Description |
(Optional) Status description. Normally describes the current state of the status.
|
state State |
(Required) Options: notSet , error , failed , notApplicable , pending , succeeded Default value: notSet |
useDefined Use defined id |
(Optional) If set, overrides the value from System.PullRequest.PullRequestId
|
pullRequestId Pull Request Id |
(Optional) If no id is given, the value from System.PullRequest.PullRequestId is taken. If a value is given, this overrides the value from System.PullRequest.PullRequestId Default value: $(System.PullRequest.PullRequestId) |
whenState When current status is in state |
(Optional) Only update the state of the status when the existing state is one of these Options: notSet , error , failed , notApplicable , pending , succeeded
|
Examples
Create and update status
steps:
- task: PullRequestStatus@0
displayName: 'Initialize status'
inputs:
name: 'my-custom-check'
action: 'Create'
state: 'pending'
description: 'Awaiting my custom check'
- script: 'echo Do something'
- task: PullRequestStatus@0
displayName: 'Update status'
inputs:
name: 'my-custom-check'
action: 'Update'
state: 'succeeded'
description: 'Check passed'
Conditionally update status
A status can be conditionally updated by using the whenState
argument. This will first check that the current status is in one of the given states before it updates.
For the example below, it will only update the status if it is in one of the following states:
- task: PullRequestStatus@0
displayName: 'Initialize status'
inputs:
name: 'my-custom-check'
action: 'Create'
state: 'pending'
description: 'Awaiting my custom check'
- script: 'echo Do something. It might even update the status'
- task: PullRequestStatus@0
displayName: 'Update status'
inputs:
name: 'my-custom-check'
action: 'Update'
state: 'succeeded'
description: 'Check passed'
whenState: 'error, failed, pending'
🐞 Known issues
If using classic pipelines and you get the error:
TF400813: The user '' is not authorized to access this resource.
Ensure Allow scripts to access the OAuth token
is checked under options. See the docs for more info.
Pull Request Description
Update pull request descriptions during pipeline runs
YAML Snippet
- task: PullRequestDescription@0
inputs:
action: append #The action to perform
content: #The content to append or replace original description with. Required when action is `append` or `replace`. For Markdown syntax, see [Syntax guidance for basic Markdown usage](http://go.microsoft.com/fwlink/?LinkId=823918) **Supports usage of variables.**
keepAppendedContent: false #Will keep the content appended from other runs of the task. This will append the current content below the existing content. Applies to action `append`
useDefined: false #Use the pre-defined id for the pull request. If set, overrides the value from `System.PullRequest.PullRequestId`. Default: `false`
pullRequestId: $(System.PullRequest.PullRequestId) #If no id is given, the value from `System.PullRequest.PullRequestId` is taken. If a value is given, this overrides the value from `System.PullRequest.PullRequestId`
outputVariable: PullRequest.DescriptionContent #The name of the output variable to write the description to. Default `PullRequest.DescriptionContent`. Applies to actions `view`
isOutput: #If set, `outputVariable` is set as output and accessible from other jobs. Applies to actions `view`
stripIdentifiers: false #Strip internal modifiers before setting variable. Applies to actions `view`
Arguments
Argument |
Description |
action Action |
(Required) The action to perform Options: append , replace , view Default value: append |
content Content |
(Required) The content to append or replace original description with. Required when action is append or replace . For Markdown syntax, see Syntax guidance for basic Markdown usage Supports usage of variables.
|
keepAppendedContent Keep appended content |
(Optional) Will keep the content appended from other runs of the task. This will append the current content below the existing content. Applies to action append
|
useDefined Use defined id |
(Optional) Use the pre-defined id for the pull request. If set, overrides the value from System.PullRequest.PullRequestId . Default: false
|
pullRequestId Pull Request Id |
(Optional) If no id is given, the value from System.PullRequest.PullRequestId is taken. If a value is given, this overrides the value from System.PullRequest.PullRequestId Default value: $(System.PullRequest.PullRequestId) |
outputVariable Output Variable |
(Optional) The name of the output variable to write the description to. Default PullRequest.DescriptionContent . Applies to actions view Default value: PullRequest.DescriptionContent |
isOutput Is output |
(Optional) If set, outputVariable is set as output and accessible from other jobs. Applies to actions view
|
stripIdentifiers Strip Identifiers |
(Optional) Strip internal modifiers before setting variable. Applies to actions view
|
Examples
Append content
Append will append content to the end of the PR on the first run. On sequential runs the content will be replaced. A markdown comment will be added to the pull request so the task knows where to replace content from.
steps:
- task: PullRequestDescription@0
inputs:
action: 'append'
content: 'This content will be appended to the bottom of the PR description'
Replace content
The following configuration will replace the entire pull request description on each run.
steps:
- task: PullRequestDescription@0
inputs:
action: 'replace'
content: 'This content will be the only part of the PR description'
Set as variable
Write the content of description to a variable.
steps:
- task: PullRequestDescription@0
name: setDescriptionVariable
inputs:
action: 'view'
outputVariable: 'PullRequest.DescriptionContent'
isOutput: true
stripIdentifiers: false
- script: echo $(setDescriptionVariable.PullRequest.DescriptionContent)
PullRequestDescription
uses some interal modifiers to know where to append content to. If you wish to remove these before setting the variable, set stripIdentifiers
to true.
Other
Setting the environment variable PRU_DESC_LOG=true
will write the old description to the log. This is mostly used during development and testing, but might be nice to know.