codigo-generator
🚀 Codigo Generator – What is it?
- Codigo Generator automates the creation of base files for your projects, combining traditional and AI-powered code generation.
- It helps you set up your project’s structure quickly, so you can focus on building features instead of repetitive setup.
- Particularly useful when starting a new project or adding a new feature (with new tables, for example).
How does it work?
- It takes a database schema and generates multiple files according to your needs.
- If you want, Codigo Generator can also enhance those files using AI for even more automation!
What kind of files does it generate?
- Mostly models, repositories, services, controllers, and more—depending on your technology stack.
What languages are supported?
- TypeScript, PHP, Java, Python, C#… and hopefully more to come!
Any frameworks?
- Yes! Check out the list below for supported frameworks and integrations.
Demo
Demo on youtube
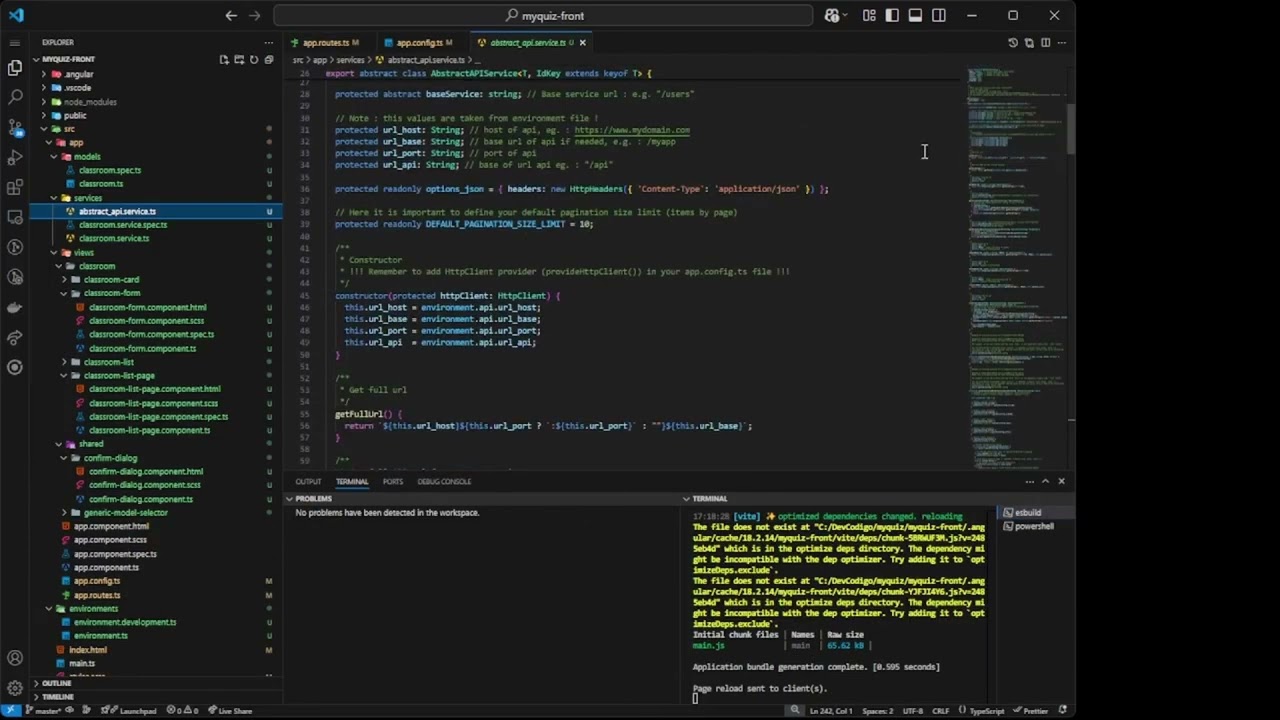
Demo with php template and symfony processors
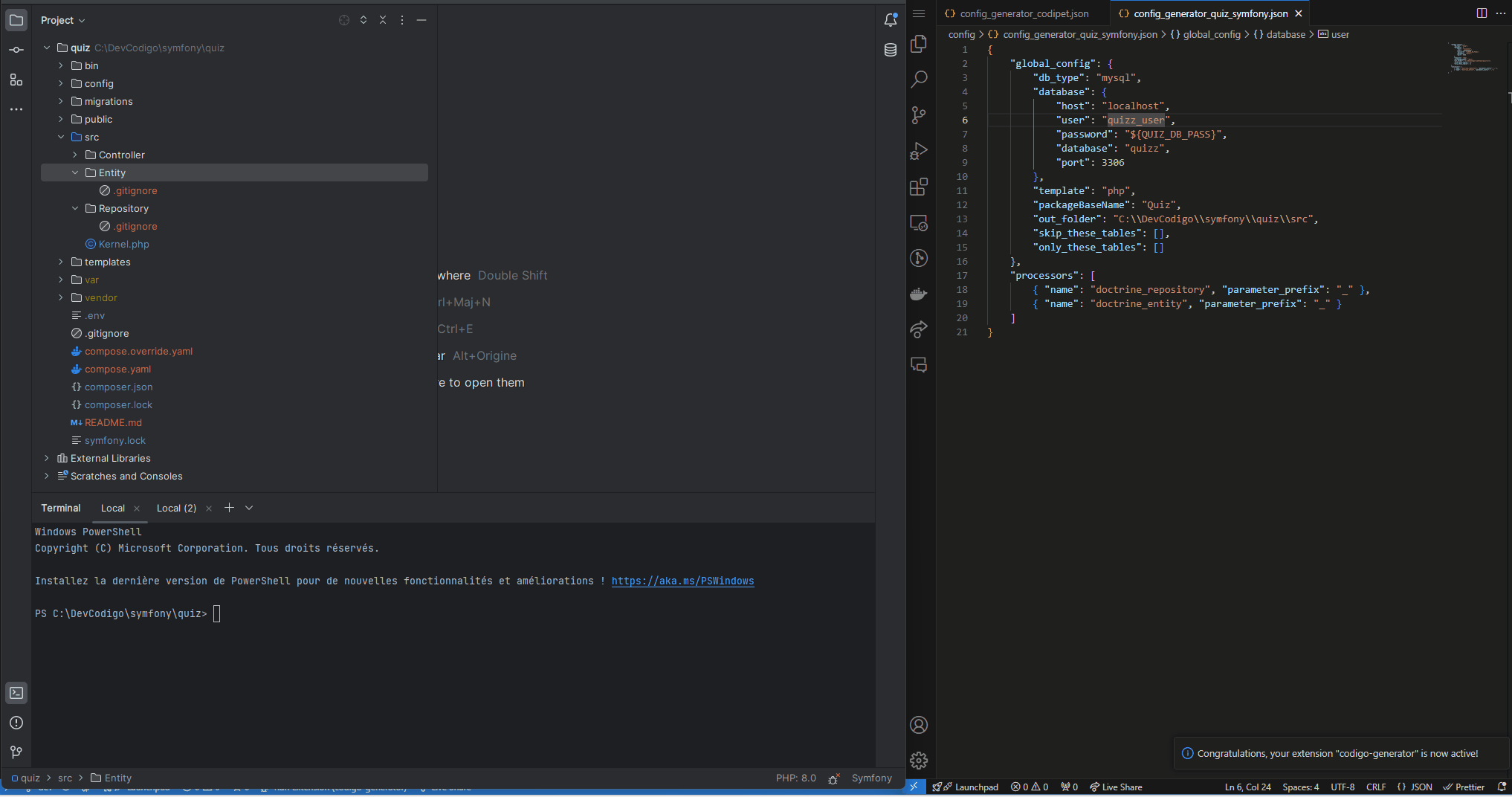
README translations
Find your README here
Requirements
You will need at least :
- A
.env
file in your project root folder as codigo-generator will load environment variables through dotenv and this file. We expect to have secret data in this file. For example :
MYSQL_DB1_ROOT_PASSWORD="OnceUponAMidnightDreary"
MYSQL_DB2_ROOT_PASSWORD="WhileIPondered"
- A json config file. You will say in that file what key you want to use for the password.
Example :
{
"global_config": {
"db_type": "mysql",
"database": {
"host": "localhost",
"user": "quizuser",
"password": "${MYSQL_DB1_ROOT_PASSWORD}",
"database": "quiz",
"port": 3306
},
"useAI": {
"enabled": true,
"apiKey": "${CHATGPT_APIKEY}"
},
"template": "php",
"packageBaseName": "MyApp",
"out_folder": "./out"
},
"processors": [
{ "name": "doctrine_repository" },
{ "name": "doctrine_entity" }
]
}
Note : Using this method is not mandatory, you could use a plain password but we strongly recommend you to use a .env
file as a good practice. So, you will be able to commit the config file if needed.
Config file
Note that you can add an optional description
property in config file. This property is not used by codigo-generator
but can help you explain the config file to others developers or whatever.
Configuration examples
Global Configuration
The global_config key contains global configuration.
db_type
db_type define the database type, it can have these values :
database
database contains database information to connect to database. You must provide these information :
- host
- user
- password
- port
- schema [optional] define the schema name to use. public by default. Not used in mysql/mariaDB Databases.
- many_to_many_sep [optional] define the string separator between both tables in a ManyToMany pivot table. "_has_" as default.
useAI
useAI is optional. It contains information for using AI service to enhance the completion of files generated by codigo-generator. Note that while codigo-generator is very fast, using AI can significantly increase processing time. However, the added value often makes it worthwhile. Check for AI column on processors list to see if processor can use AI.
Careful, when you use AI, you may need to make some changes to the code. That's fair enough.
If you want to use AI, you must provide the following information:
- apiKey: Your AI service API key. It is strongly recommended to use a placeholder (e.g.,
${CHATGPT_APIKEY}
) and store this key in an environment file!
- enabled: A boolean value to activate or deactivate AI. The default is
false
.
- aiName: [Optional] The name of AI Service to use. By default, we use the OpenAI service. Here are the values you can use for aiName :
- OpenAI : OpenAI API service
- DeepSeek : DeepSeek API service
- Mistral : Mistral AI API service
- duration_warning: [Optional] Time limit in seconds (e.g. "duration_warning": 20) before triggering a warning for an AI process taking too long. The warning indicates the elapsed time and suggests adjusting the settings.
Additional properties
These additional properties are optional. Be cautious when using them, as they can further increase processing time.
- model: The name of the AI model for your AI Service. If it is not provided, we use a default value. The default value depends of the AI service you use.
- OpenAI : gpt-4o
- DeepSeek : deepseek-chat
- Mistral : ministral-8b-latest
- temperature: Defines the temperature for AI requests. Not send if not provided.
- max_completion_tokens: Sets the maximum number of tokens for AI completions. Not send if not provided.
Here’s some configuration examples :
"useAI": {
"enabled": true,
"model": "gpt-4o-mini",
"apiKey": "${CHATGPT_APIKEY}"
}
"useAI": {
"enabled": true,
"aiName": "DeepSeek",
"apiKey": "${DEEPSEEK_APIKEY}"
}
"useAI": {
"enabled": true,
"aiName": "Mistral",
"model": "mistral-small-latest",
"apiKey": "${MISTRAL_APIKEY}"
}
template
template define the language mostly (well, so far), it can have these values :
- typescript
- php
- java
- python
- csharp
packageBaseName
packageBaseName is optional but necessary for some templates, as this is your base package/namespace name.
If you use the model processor with php template and "packageBaseName": "MyApp"
, your model files will contain namespace MyApp\Models;
.
If you use the model processor with java template and "packageBaseName": "com.myapp"
, your model files will contain package com.myapp.models;
.
If you use the model processor with csharp template and "packageBaseName": "AngularApp1.Server"
, your model files will contain namespace AngularApp1.Server.Models;
.
django processors need packageBaseName
, this is the name of the app.
out_folder
out_folder is where the files must be created. Codigo-generator generate files based on your config file, so yes, there are gonna be files generated, and there are gonne be there. This path value can be :
- a relative path to this workspace, your relative path must start with a dot, e.g. =>
"out_folder": "./out"
- an absolute path =>
"out_folder": "C:\\Users\\marco\\OneDrive\\tmp\\out"
skip_these_tables
skip_these_tables is optional. It is an array of table names to skip.
Example : "skip_these_tables": [ "classrooms" ]
This example will make the classrooms table skipped from the process.
only_these_tables
only_these_tables is optional. It is an array of table names that will be exclusively processed.
Example : "only_these_tables": [ "classrooms", "users" ]
This example will make the classrooms and users table processed but not the others. Note that if classrooms was in skip_these_tables array too, it would be skipped (fair, isn't it ?).
Processors
The processors key contains an array of processors. Every processor has a job to do... for you.
Every processor must have these information :
name : the name of the processor (see below for a list of available processor names)
generate : is optional. It's a boolean value, true means processor will work, false he will be skipped. Default to true.
parameter_prefix : is optional.. This is the text to append to function parameters. By default it is 'r'.
- Example :
function sayHello(rName: string) { ... }
- with this config
"parameter_prefix": "_"
we will have : function sayHello(_Name: string) { ... }
custom_base_template : is optional. It is the absolute path of your custom template file if you want something special. You should not use this if it's not necessary. See below for more information.
extra_config : is optional. It is a json with custom configuration that will work only for some processors. See below for more information.
useCamelCaseForClass : is optional. true to use camelCase for class names, false otherwise. Default is true.
useCamelCaseForField : is optional. true to use camelCase for field names, false otherwise. Default is true. Note that some processors might ignore this property due to language normalization.
Processor list
Here is a list of available processors for every templates. Hope it will grow in time.
Some processors can generate extra files.
typescript template
name |
details |
extra files |
AI ? |
model |
Model class |
|
|
angular_service |
Angular Service class to call back-end api |
'abstract_api.service.ts' |
yes |
angular_view_model_form |
Angular form view component for model |
angular_generic_model_selector component |
yes |
angular_view_model_card |
Angular card view component for model |
|
yes |
angular_view_model_list |
Angular list view component for model |
'angular_confirm_dialog' component |
yes |
angular_view_model_list_page |
Angular page to show list view component |
|
|
ionic_angular_service |
Ionic Angular Service class to call back-end api |
'abstract_api.service.ts' |
yes |
ionic_angular_view_model_form |
Ionic Angular form view component for model |
angular_generic_model_selector component |
yes |
ionic_angular_view_model_card |
Ionic Angular card view component for model |
|
yes |
ionic_angular_view_model_list |
Ionic Angular list view component for model |
'angular_confirm_dialog' component |
yes |
ionic_angular_view_model_list_page |
Ionic Angular page to show list view component |
|
|
row_mapper |
Rowmapper class, like a model class, but only with relations with childs |
|
|
loopback_model |
loopback framework class model |
|
|
loopback_repository |
loopback framework class repository |
|
|
loopback_service |
loopback framework class service |
|
yes |
loopback_controller |
loopback framework class controller |
|
yes |
postman |
Postman collection (will be on root) |
|
|
php template
name |
details |
extra files |
AI ? |
model |
Model class |
|
|
pdo_dao |
Dao (repository) class with PDO |
|
|
doctrine_entity |
Doctrine Entity for Symfony users (php 8) |
|
|
doctrine_repository |
Doctrine Repository for Symfony users (php 8) |
|
|
artisan_make_model |
Generate a single bash file with artisan make commands |
|
|
eloquent_model |
Eloquent model file for Laravel users |
|
|
symfony_service |
Symfony Service |
|
|
symfony_api_controller |
Symfony api Controller |
|
|
postman |
Postman collection (will be on root) |
|
|
java template
name |
details |
extra files |
AI ? |
model |
Model class |
|
|
jpa_entity |
Spring JPA Entity |
|
|
jpa_repository |
Spring JPA Repository |
|
yes |
spring_service |
Spring service using repository |
|
yes |
spring_controller |
Spring controller using service |
|
yes |
spring_security_jwt |
Spring security with JWT and single role |
|
|
postman |
Postman collection (will be on root) |
|
|
python template
name |
details |
extra files |
AI ? |
django_model |
Django model class |
|
|
django_import_models |
One models.py file to import your models, will be on root |
|
|
django_controller |
Django controller class |
|
|
django_controller_path |
One urls_controllers.py file to use your controllers, will be on root |
|
|
postman |
Postman collection (will be on root) |
|
|
csharp template
name |
details |
extra files |
AI ? |
model |
model class |
|
|
efcore_model |
EF Core model class (prefer using efcore to generate models) |
|
|
efcore_repository |
EF Core repository |
IAbstractRepository/AbstractRepository |
|
net_service |
.Net service |
|
|
net_controller |
.Net controller class |
|
|
net_program |
.Net Program.cs file with import for services/repositories |
|
|
postman |
Postman collection (will be on root) |
|
|
custom_base_template
custom_base_template is the absolute path of your custom template directory if you want something special.
You should not use this unless it is necessary.
Processors use template files to populate data. Every template receives incoming data delimited by {{ }}. If you use your own, you must know what it will receive.
You can check the templates in the templates
folder on the GitLab extension repository.
Example:
Let's say you want your own template file for the java
template and model
processor. First, create a local folder to store your template file. For example: "c:/my_templates". Then go to the GitLab project repository templates
folder, then in the java
folder.
There you will find two files related to the model
processor (there could be one or more):
- tpl_model_enum.java
- tpl_model.java
Download these two files to your "c:/my_templates" folder but don't rename them!
Change their contents to suit your needs. Be careful with {{ }} data.
Then you're good to go. You can run your processor, and it will use your files.
extra_config as said before can be use only with some processors.
Example with an extra config :
"processors": [
{ "name": "model", "generate": false },
{ "name": "jpa_entity", "extra_config": { "lombok": false } },
{ "name": "jpa_repository", "generate": false }
]
This is the list of processors and their available extra_config
template |
name |
extra_config property |
default value |
Details |
Example |
java |
model |
lombok |
true |
Add lombok annotations |
"extra_config": { "lombok": false } |
java |
jpa_entity |
lombok |
true |
Add lombok annotations |
"extra_config": { "lombok": false } |
java |
jpa_entity |
use_enum |
true |
Use enum class when enum |
"extra_config": { "use_enum": false } |
java |
spring_service |
logger |
true |
Add logger |
"extra_config": { "logger": false } |
java |
spring_controller |
logger |
true |
Add logger |
"extra_config": { "logger": false } |
typescript |
loopback_repository |
datasource_name |
schema name |
DataSource name for repository |
"extra_config": { "datasource_name": "MyApp" } |
typescript |
ionic_angular_service |
message_service |
false |
Set to true to add an AppMessageService component (need @ngx-translate) |
"extra_config": { "message_service": true } |
php |
artisan_make_model |
option |
empty string |
Artisan model command option |
"extra_config": { "option": "--all" } |
php |
eloquent_model |
do_getters_setters |
false |
Add Getters/Setters |
"extra_config": { "do_getters_setters": true } |
php |
symfony_api_controller |
base_api |
"/api" |
Base for api url |
"extra_config": { "base_api": "/myapi" } |
php |
symfony_api_controller |
folder_api |
"Api" |
Folder name where to put the files |
"extra_config": { "folder_api": "MyApi" } |
java |
spring_security_jwt |
user_field/pass_field/role_field |
|
You must name fields used to identify user (email, username, ..), password (pass, password, ...) and role (rolename, role, ...) |
"extra_config": { "user_field": "users.email", "pass_field": "users.password", "role_field": "roles.role" } |
postman processors
Those are extra_config properties available for postman
processors.
with_base_api can let you define if your api has a main base name. For example /api
. As a best practice, this would be added as a {{base_api}}
environment variable in your postman collection.
"extra_config": { "with_base_api": false }
name can let you defined filename of postman file generated by the processor. Default to default-postman
. For java, he can have package name.
"extra_config": { "name": "myproject-postman" }
use_model_for_api_endpoint
use_model_for_api_endpoint is an extra_config property available for processors making files with api path (e.g. postman, controller, angular services, etc.).
It has a default value of false
.
Behavior is :
- true : base model path uses table name. e.g. : /users
- false : base model path uses model name. e.g. : /user
standalone for Angular
standalone is an extra_config property available for Angular processors to ask for creating standalone components. Default to true.
Extension Settings
No extension settings by now.
Author
Marc Flausino
Contributors