Template Generator
Template Generator is a powerful extension for Visual Studio Code that simplifies the creation and sharing of file templates with an integrated context system. Save time by quickly generating repetitive files and project structures tailored to your specific needs.
Create the configuration
On your project:
- Create a folder
vscode_templates
- On
vscode_templates
folder, create a config.json
file
config.json
[
{
"description": "Generate CRUD mocha/chai TEST",
"context": [
{
"variable": "route",
"description": "Route URL",
"type": "string",
"placeholder": "dogs"
},
{
"variable": "describe",
"description": "Test describe",
"type": "string",
"placeholder": "DOG"
},
...
],
"files": [
"src/tests/__VSCCONTEXT__describe__.test.ts",
...
]
},
...
]
Key |
Description |
description |
Description presented as a choice in the template generation UI |
context |
Array of context objects providing information for template variables |
- variable |
Name of the context variable |
- description |
Description of the context variable shown in the UI |
- type |
Type of the context variable (string or number) |
- placeholder |
Placeholder value displayed in the input field |
files |
Array of file paths representing files to parse, located outside the context |
File Paths:
- Project:
/src/tests/...
- config.json:
generate_template/src/tests/...
During generation, files are copied from the generate_template
structure.
File paths support context substitution:
src/tests/__VSCCONTEXT__route__.ts
: Build path with the route
context value as the filename.
src/tests/dog.test.ts
: Build path with the filename directly.
src/tests/__VSCCONTEXT__route__/another/__VSCCONTEXT__route__/file.test.ts
: Builds path using context route
value as path, and file.test.ts
as the filename.
src/tests/__VSCCONTEXT__route__/another/__VSCCONTEXT__route__/__VSCCONTEXT__describe__.ts
: Build path using context with route
context value as path, and describe
context value as the filename.
Create a template
src/tests/__VSCCONTEXT__describe__.test.ts
declare var agent: any;
import { expect } from "chai";
import { describe, it } from "mocha";
import chai from 'chai';
import chaiHttp from 'chai-http';
chai.use(chaiHttp);
describe('__VSCCONTEXT__describe__ Validation', async function () {
let dogId: string;
let res: any;
let bodyPost = {
"name": "Rex",
"age": "12"
}
it('it should CREATE a __VSCCONTEXT__describe__', async () => {
try {
res = await agent.post('/__VSCCONTEXT__route__/').send(bodyPost)
expect(res).to.have.status(200);
dogId = res.body;
} catch (err) {
console.error(res.body);
throw err;
}
});
it('it should UPDATE test __VSCCONTEXT__describe__', async () => {
try {
res = await agent.patch('/__VSCCONTEXT__route__/' + dogId).send({ name: "rex-update" })
expect(res).to.have.status(200);
} catch (err) {
console.error(res.body);
throw err;
}
});
it('it should GET one __VSCCONTEXT__describe__', async () => {
try {
res = await agent.get('/__VSCCONTEXT__route__/' + dogId);
expect(res).to.have.status(200);
} catch (err) {
console.error(res.body);
throw err;
}
});
it('it should DELETE test __VSCCONTEXT__describe__', async () => {
try {
res = await agent.delete('/__VSCCONTEXT__route__/' + dogId);
expect(res).to.have.status(200);
} catch (err) {
console.error(res.body);
throw err;
}
});
});
To add a context, you need to set __VSCCONTEXT__{CONTEXT_KEY}__
, for example :
__VSCCONTEXT__route__
You can set something before or after the context, for example :
__VSCCONTEXT__route__/something
something__VSCCONTEXT__route__
something__VSCCONTEXT__route__/something
Auto completion
The extension provides an auto completion feature for contexts. The displayed contexts are those detected in the configurations that include the currently edited file.
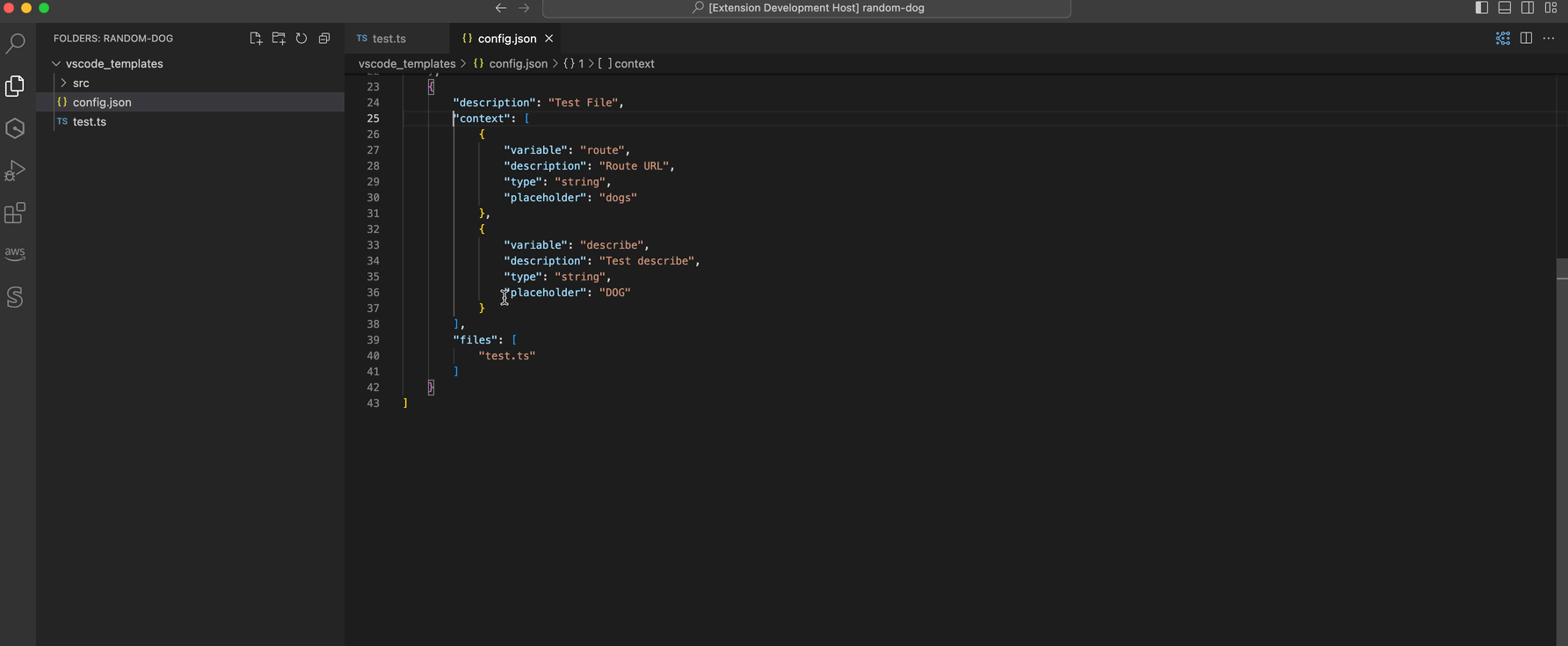
Multi project workspace support
The extension supports workspaces with multiple projects, by first suggesting the project before proposing the project's template to be generated.
Generate template
- Choose
Template generator
with CTRL+P
- Choose the template that you want
If there is no context in your template, the form will not be displayed; the template will be generated automatically.
Demo
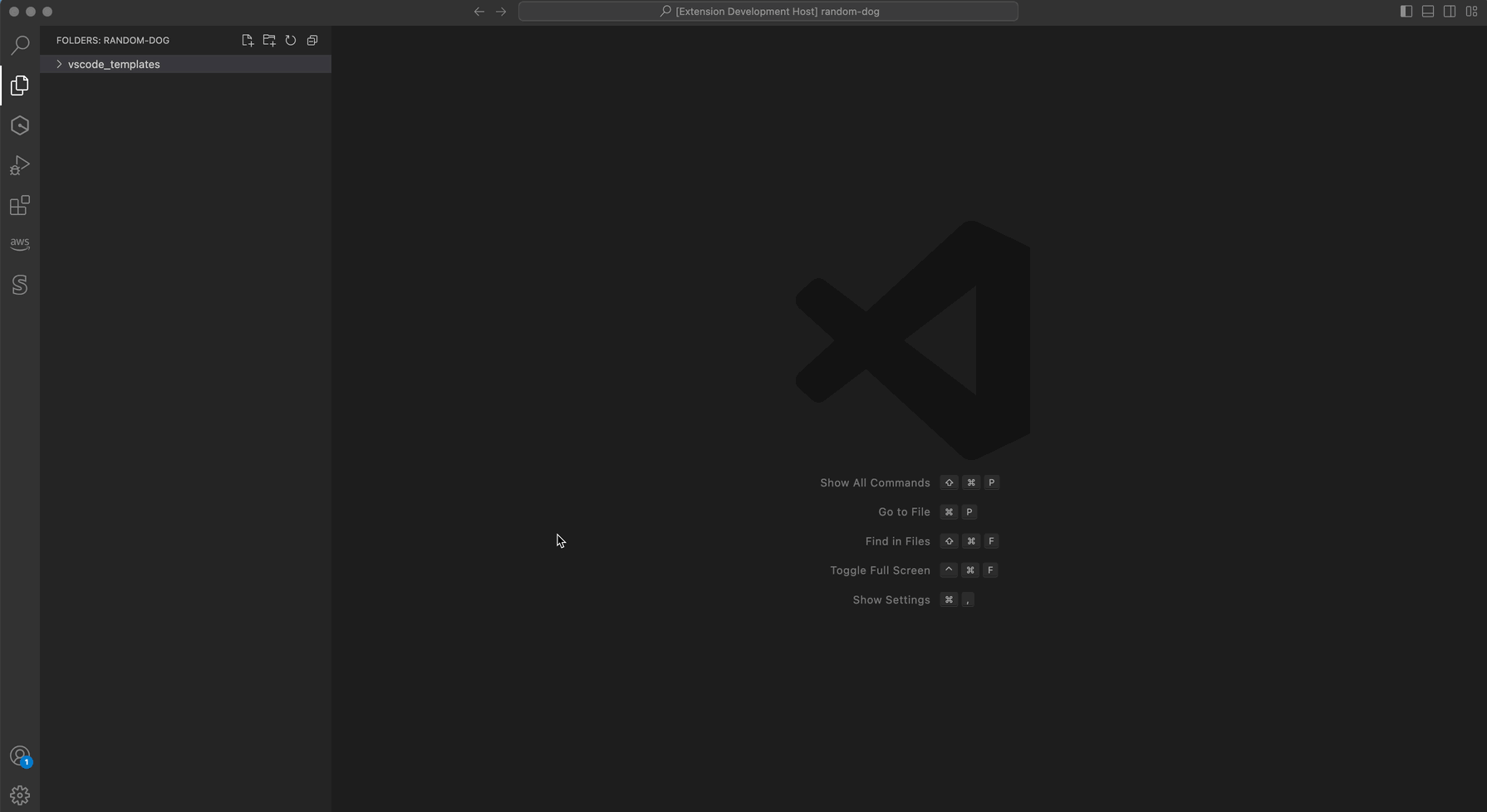