Magento 2 Snippets Extension for Visual Studio Code
Overview
Welcome to the Magento 2 Snippets extension for Visual Studio Code! This extension is designed to streamline the development process in Magento 2 by providing a collection of code snippets that simplify common tasks. Whether you're a beginner or an experienced Magento 2 developer, these snippets will save you time and boost your productivity.
Installation
- Open Visual Studio Code.
- Access the Extensions view by clicking on the square icon in the sidebar or by pressing
Ctrl+Shift+X
.
- Search for "Magento2 snippets."
- Click on "Install" to install the extension.
- Once installed, you can start using the snippets in your Magento 2 projects.
Usage
Using Magento 2 Snippets is straightforward. Follow these steps to start using the snippets in your code:
- Open a Magento 2 project in Visual Studio Code.
- Create or open a PHP, XML, or PHTML file.
- Type the prefix of a snippet and press
Tab
to trigger the code snippet.
For example, to pick up a product by ID in Magento 2, type m2.get_product_by_id
and press Tab
. The extension will automatically generate the code structure for you.
Trigger: m2.get_product_by_id
Output:
$objectManager = \Magento\Framework\App\ObjectManager::getInstance();
$productId = 1; // Replace with the desired product ID
$product = $objectManager->create('\Magento\Catalog\Model\Product')->load($productId);
echo $product->getName();
// note: Magento does not recommend using ObjectManager in production
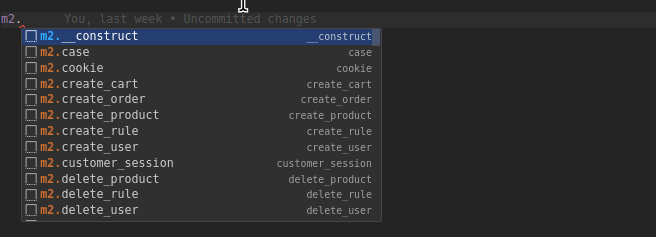
Available Snippets
Here is a list of some of the available snippets:
m2.routes
: Create a routes file.
m2.layout.block
: Create a new block class.
m2.logger
: Create a log file.
m2.object_manager
: Create an object manager.
m2.cls_product
: Factory class for products in Magento.
m2.cls_category
: Factory class for categories in Magento.
m2.list_carts
: List all shopping carts.
m2.create_order
: Create a new order.
m2.get_order_by_id
: Get an order by ID.
m2.get_base_url
: Get base URL.
m2.helper
: Get Helper.
See all available commands.
m2.get_block_html
: Get Block HTML.
m2.log_custom
: Log custom message.
m2.log_json_encode
: Log JSON encoded data.
m2.customer_session
: Handle customer session.
m2.new_session_catalog
: Create and handle catalog session.
m2.new_session_customer
: Create and handle customer session.
m2.new_session_checkout
: Create and handle checkout session.
m2.list_products
: List all products.
m2.list_users
: List all users.
m2.list_rules
: List all sales rules.
m2.create_product
: Create a new product.
m2.create_user
: Create a new user.
m2.create_rule
: Create a new sales rule.
m2.get_product_by_id
: Get a product by ID.
m2.get_user_by_id
: Get a user by ID.
m2.get_rule_by_id
: Get a sales rule by ID.
m2.update_product
: Update a product.
m2.update_user
: Update a user.
m2.update_rule
: Update a sales rule.
m2.delete_product
: Delete a product.
m2.delete_user
: Delete a user.
m2.delete_rule
: Delete a sales rule.
m2.list_orders
: List all orders.
m2.get_core_config_data
: Get core configuration data.
m2.set_core_config_data
: Set core configuration data.
m2.create_cart
: Create a shopping cart.
m2.list_carts
: List all shopping carts.
m2.layout.args
: Add arguments.
m2.layout.arg
: Add an argument.
m2.layout.attr.translate
: Translate attribute.
m2.layout.attr.xsi:type
: xsi:type attribute.
m2.layout.attr.handle
: Handle attribute.
m2.layout.attr.destination
: Destination attribute.
m2.layout.attr.element
: Element attribute.
m2.layout.attr.htmlId
: htmlId attribute.
m2.layout.attr.htmlClass
: htmlClass attribute.
m2.layout.attr.htmlTag
: htmlTag attribute.
m2.layout.attr.output
: Output attribute.
m2.layout.attr.label
: Label attribute.
m2.layout.attr.as
: As attribute.
m2.layout.attr.template
: Template attribute.
m2.layout.attr.class
: Class attribute.
m2.layout.attr.name
: Name attribute.
m2.layout.attr.before
: Before attribute.
m2.layout.attr.after
: After attribute.
m2.layout.attr.cacheable
: Cacheable attribute.
m2.layout.attr.display
: Display attribute.
m2.layout.attr.remove
: Remove attribute.
m2.layout.container
: A structure without content that holds other layout elements such as blocks and containers.
m2.layout.containerWrap
: A structure without content that holds other layout elements such as blocks and containers.
m2.layout.refContainer
: Targets containers and applies updates specified.
m2.layout.move
: Sets the declared block or container element as a child of another element in the specified order.
m2.layout.remove
: Is used only to remove the static resources linked in a page section.
m2.layout
: Magento 2 layout scaffold.
m2.layout.update
: Includes a certain layout file.
m2.module
: Magento 2 module.xml.
m2.di
: Magento 2 DI scaffold.
m2.di.pref
: Magento 2 DI preference.
m2.di.type
: Magento 2 DI type.
m2.di.plugin
: Magento 2 DI plugin.
m2.di.args
: Magento 2 DI type arguments.
m2.di.args.item
: Magento 2 DI type argument item.
m2.di.virtualtype
: Magento 2 DI virtual type.
m2.events
: Magento 2 events scaffold.
m2.events.event
: Magento 2 event.
m2.events.observer
: Magento 2 event observer.
m2.routes
: Magento 2 routes scaffold.
m2.routes.router
: Magento 2 router.
m2.routes.route
: Magento 2 route.
m2.__construct
: Constructor.
m2.echo
: 'echo' statement.
m2.foreach
: foreach(iterable_expr as $value) {...}.
m2.foreach_k
: foreach(iterable_expr as $key => $value) {...}.
m2.include
: 'include' statement.
m2.include_once
: 'include_once' statement.
m2.private_function
: private function.
m2.private_static_function
: private static function.
m2.protected_function
: protected function.
m2.protected_static_function
: protected static function.
m2.public_function
: public function.
m2.public_static_function
: public static function.
m2.require
: 'require' statement.
m2.require_once
: 'require_once' statement.
m2.throw_new
: throw new.
m2.print_r
: PHP print_r() function.
m2.try_catch
: Exceptions Block.
m2.try_catch_example
: Exceptions Block Example.
m2.globals
: $GLOBALS array.
m2.server
: $_SERVER array.
m2.request
: $_REQUEST array.
m2.post
: $_POST array.
m2.get
: $_GET array.
m2.file
: $_FILES array.
m2.env
: $_ENV array.
m2.cookie
: $_COOKIE array.
m2.session
: $_SESSION array.
m2.for_loop
: For loop.
m2.for_end
: For end loop.
m2.loop_while
: While loop.
m2.while_end
: While end loop.
m2.do_while
: Do while loop.
m2.switch_case
: Switch block.
m2.case
: Case addon block.
m2.if_ternary
: Ternary statement.
m2.if
: If block.
m2.if_else
: If else block.
Showing the magento2 version
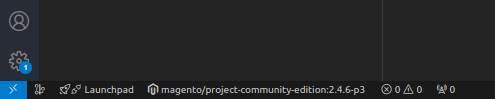
Customization
You can customize the extension to match your coding style and preferences. To do so, follow these steps:
- Go to File > Preferences > Settings.
- Search for "Magento2 snippets" in the search bar.
- You can modify existing snippets or add your own.
Contributions
This extension is open source, and contributions are welcome. If you have snippets or improvements to share, visit our GitHub repository to make a contribution.
Support
If you encounter issues, have suggestions, or need assistance, please visit the issue tracker on GitHub to report problems or request help.
Happy coding with Magento 2 Snippets in Visual Studio Code!
GitHub Repository
Report Issues
Support Contact