VSCode Macros
日本語版README
Add scripting macro functionality to your VSCode
Enhance your work efficiency and productivity by automating repetitive text editing operations.
Features
- Write macros in JavaScript
- Utilize the VSCode extension API in your macros
(Some limitations apply due to API specifications)
- Use Node.js modules in your macros
- Run macros from the command palette or with custom keyboard shortcuts
- Organize macros into separate files for easy management
- Debug macros using VSCode's built-in debugger
Demos
Write and run your macro instantly
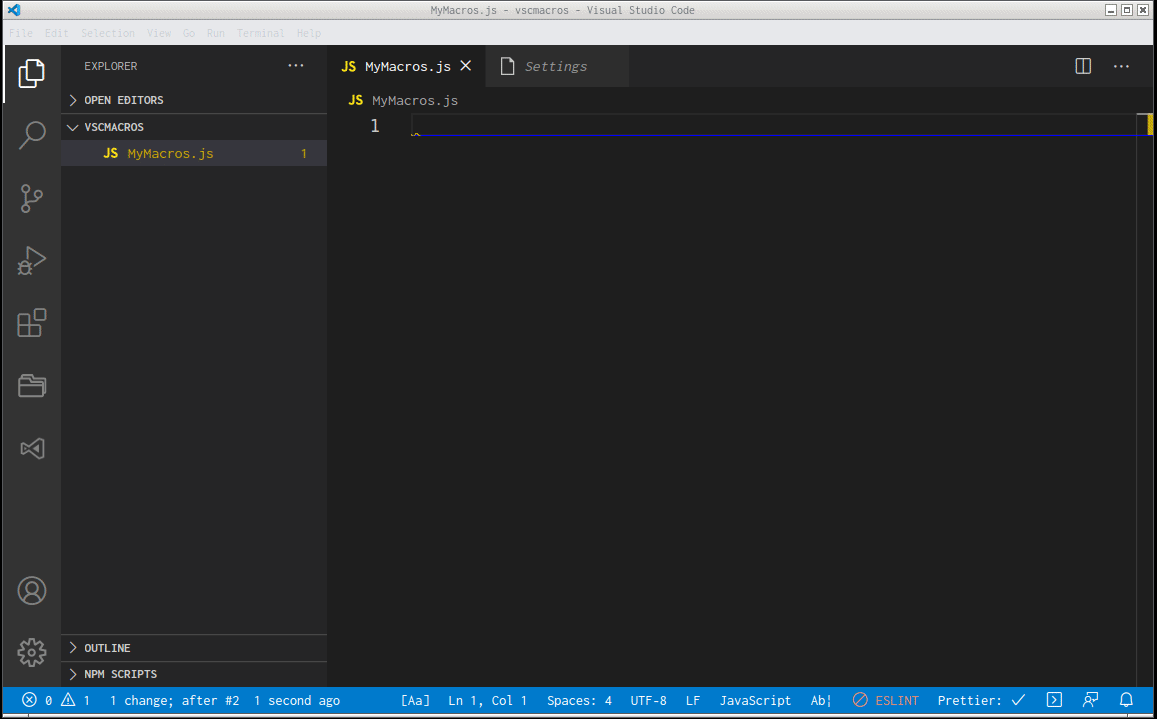
Switch between macro files and apply multiple macros in combination
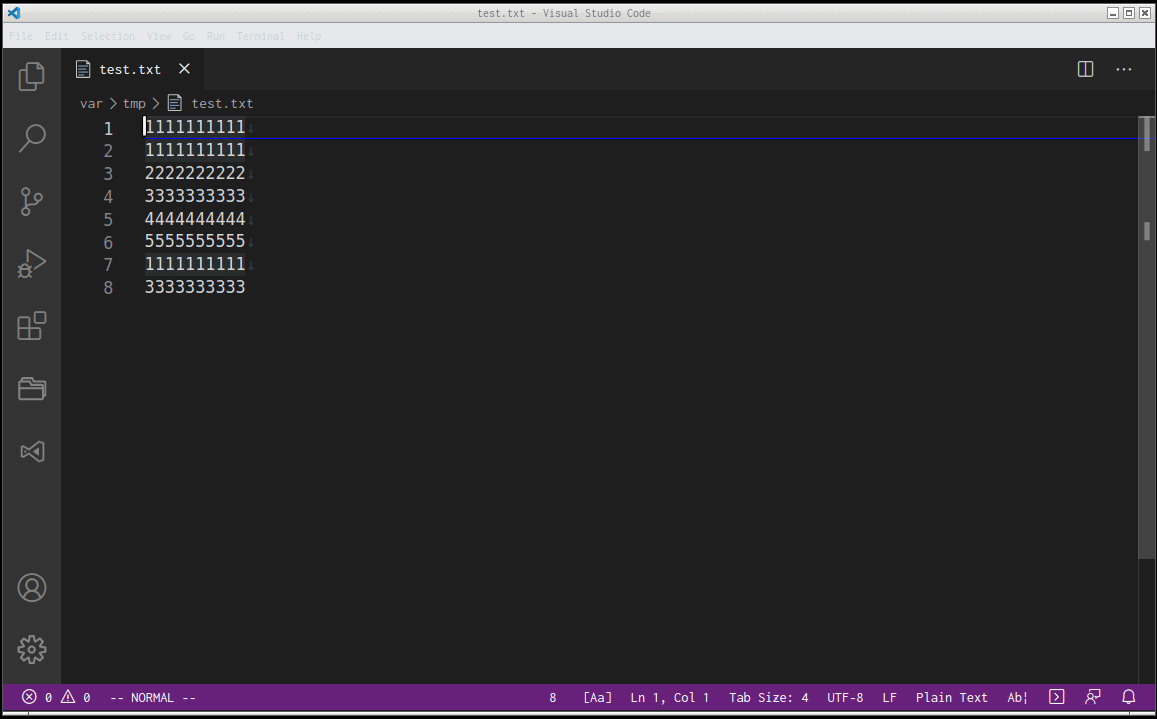
Debug your macros with VSCode's debugger
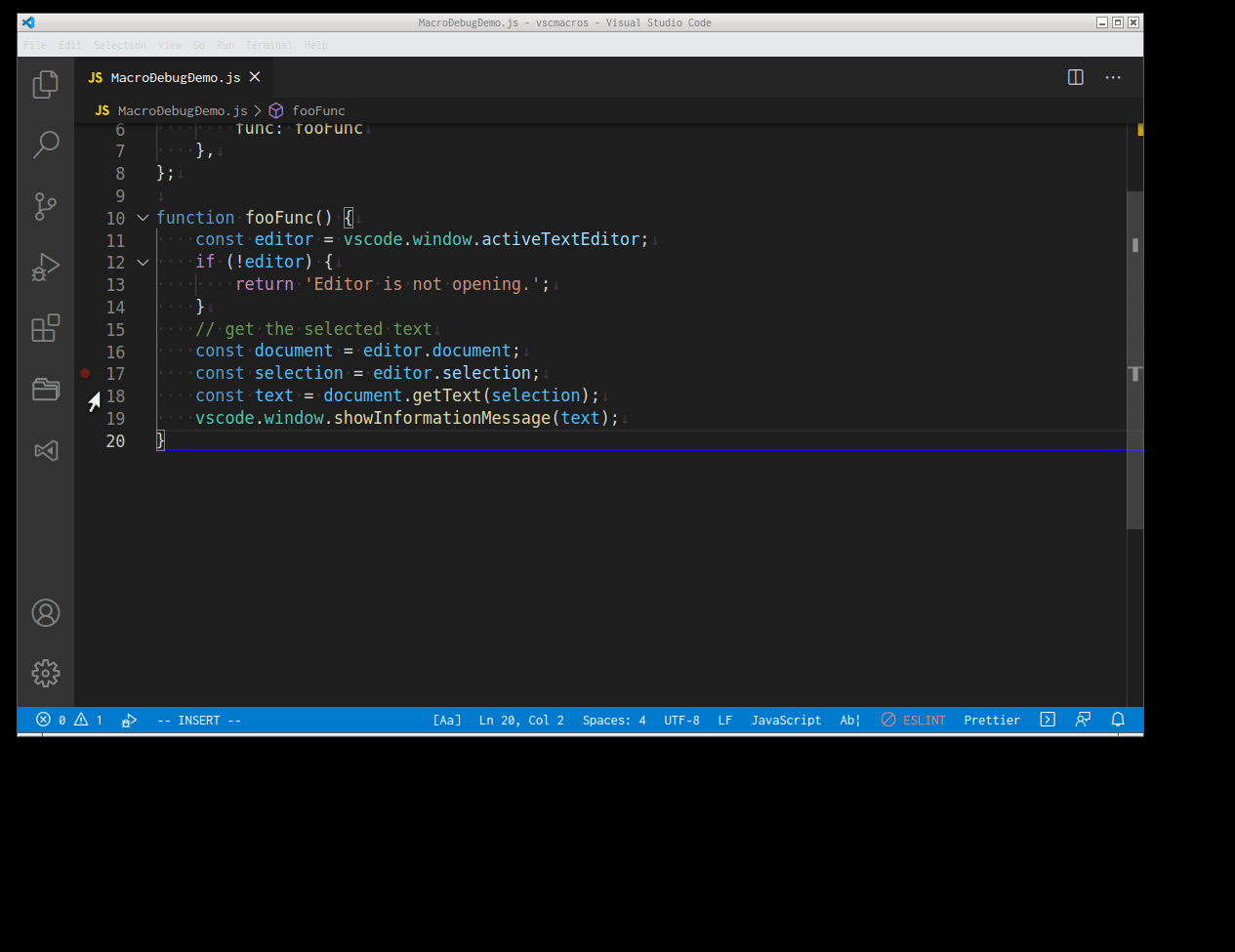
Setting up the extension
Create a folder in any location to store your macro files.
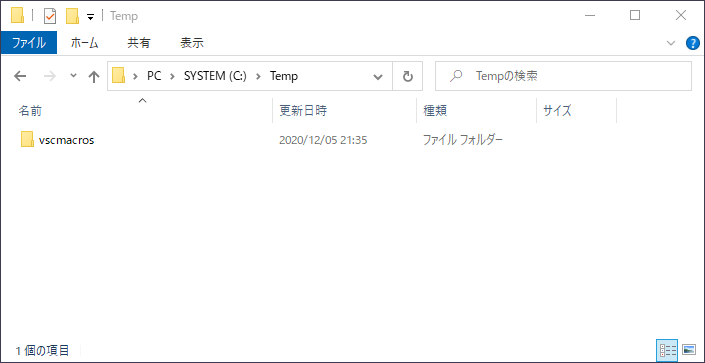
Open the folder with VSCode.
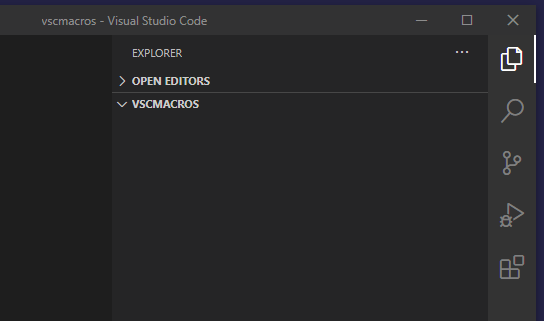
Create a new file and paste the example macro code below.
Macros are written in JavaScript(CommonJS).
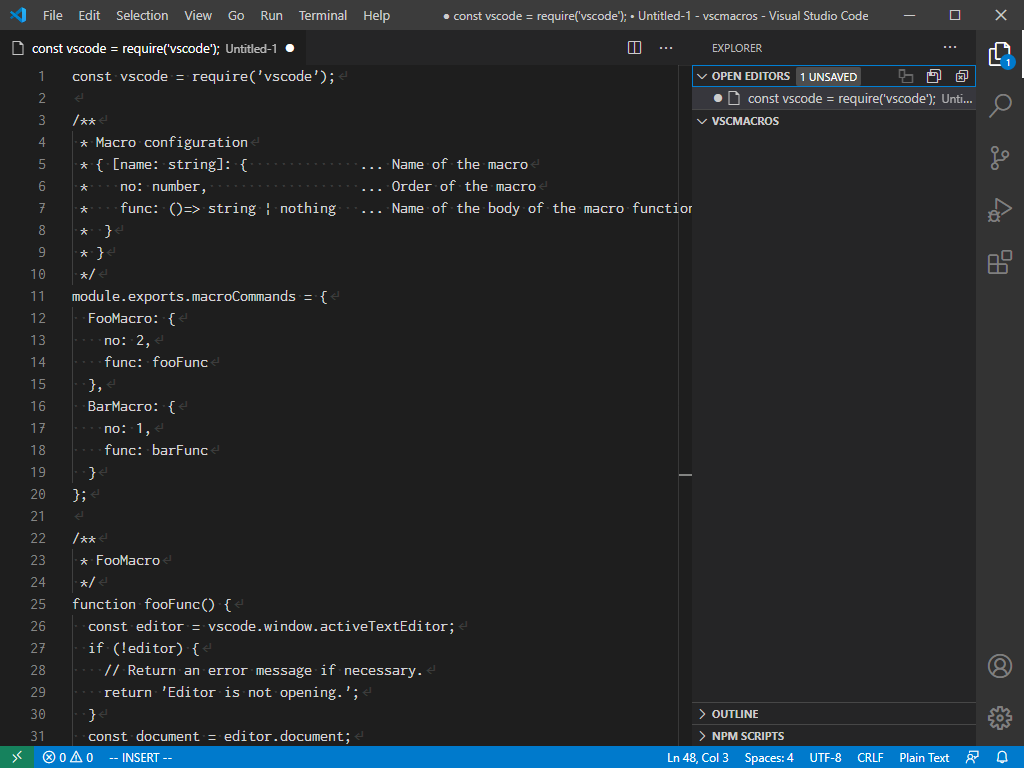
const vscode = require('vscode');
/**
* Macro configuration settings
* { [name: string]: { ... Name of the macro
* no: number, ... Order of the macro
* func: ()=> string | undefined ... Name of the body of the macro function
* }
* }
*/
module.exports.macroCommands = {
FooMacro: {
no: 2,
func: fooFunc
},
BarMacro: {
no: 1,
func: barFunc
}
};
/**
* FooMacro
*/
function fooFunc() {
const editor = vscode.window.activeTextEditor;
if (!editor) {
// Return an error message if necessary.
return 'Editor is not opening.';
}
const document = editor.document;
const selection = editor.selection;
const text = document.getText(selection);
if (text.length > 0) {
editor.edit(editBuilder => {
// To surround a selected text in double quotes(Multi selection is not supported).
editBuilder.replace(selection, `"${text}"`);
});
}
}
/**
* BarMacro(asynchronous)
*/
async function barFunc() {
await vscode.window.showInformationMessage('Hello VSCode Macros!');
// Returns nothing when successful.
}
To create a new macro quickly, you can use VSCode's snippet feature with a snippet like this:
{
"Create new VSCode macro": {
"prefix": "vscmacro",
"body": [
"const vscode = require('vscode');",
"",
"/**",
" * Macro configuration settings",
" * { [name: string]: { ... Name of the macro",
" * no: number, ... Order of the macro",
" * func: ()=> string | undefined ... Name of the body of the macro function",
" * }",
" * }",
" */",
"module.exports.macroCommands = {",
" $1: {",
" no: 1,",
" func: $2,",
" },",
"};",
"",
"/**",
" * Hello world",
" */",
"async function $2() {",
" const editor = vscode.window.activeTextEditor;",
" if (!editor) {",
" // Return an error message if necessary.",
" return 'Active text editor not found.';",
" }",
" const document = editor.document;",
" const selection = editor.selection;",
" const text = document.getText(selection);",
"",
" editor.edit((editBuilder) => {",
" editBuilder.replace(selection, `Hello world! \\${text}`);",
" });",
"}"
],
"description": "VSCode Macros Template"
}
}
Give the file a name of your choice (*.js) and save it in the macro folder.
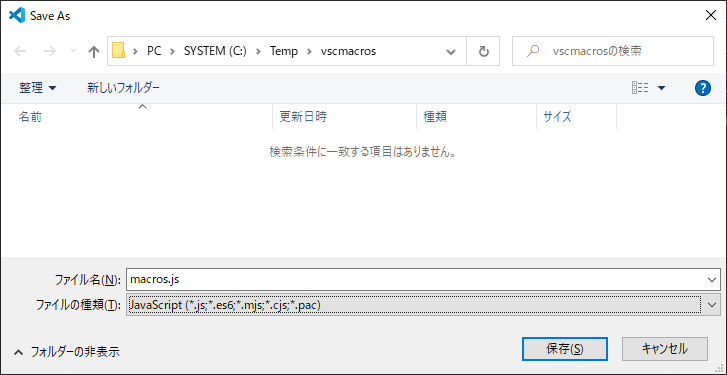
Open VSCode's settings, search for "vscode macros", and enter the macro file path in the 'Macro File Path' text box.
NOTE: Version 1.3.0 and above support multi-root, workspace, and workspace folders.
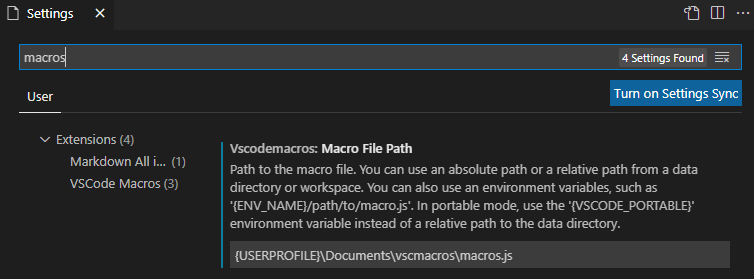
- You can use environment variables in the macro file path, such as
{ENV_NAME}/path/to/macro.js .
- For VSCode portable mode version 1.3.0 and later, use the
{VSCODE_PORTABLE} environment variable.
NOTE: When using this extension in portable mode, set the relative path to the data directory up to version 1.2.0. In version 1.3.0 and later, use the environment variable instead of the relative path.
Assigning frequently used macros to user commands
Open VSCode's settings, search for "vscode macros", then click {Edit in settings.json} in the User Macro Commands field.
NOTE: Version 1.3.0 and above support multi-root, workspace, and workspace folders.

Register the macro path and name in the JSON file as shown below. (Up to 10 commands)
"vscodemacros.userMacroCommands": [
{
"path": "C:\\Temp\\macros\\FooMacro.js",
"name": "FooMacro"
},
{
"path": "C:\\Temp\\macros\\BarMacro.js",
"name": "BarMacro"
},
{
"path": "",
"name": ""
},
{
"path": "",
"name": ""
},
{
"path": "",
"name": ""
},
],
Using your macros
You can run your macros from the command palette:
Press {F1} to open the command palette, type "run a macro", and press {Enter} .

Select the macro name from the list.

To switch to another macro file, use the "select a macro file" command.

You can assign your macros to User Macro 01 through User Macro 10 :
Press {F1} to open the command palette, then type "vscmacros".
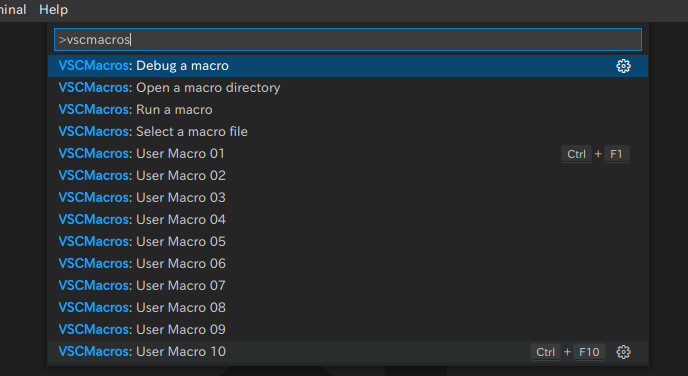
Click the {⚙} icon of the user command you want to assign a shortcut key to.

Debugging your macros
You can debug your macros in the extension development host on VSCode:
Open the macro folder with VSCode.
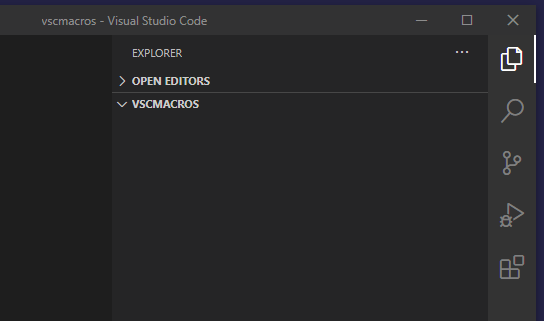
Open the macro file and set breakpoints as needed.
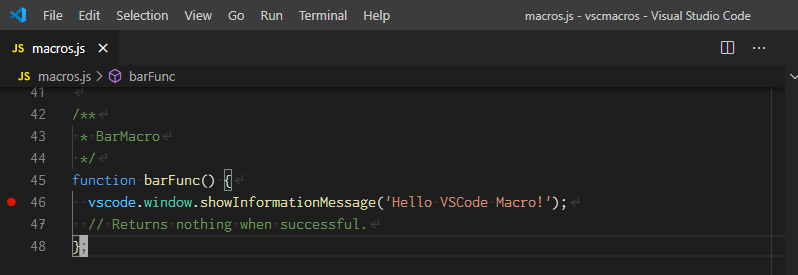
Press {F5} and select VS Code Extension Development (preview) from the environment list.
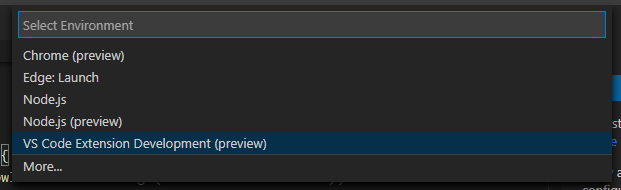
For older VSCode versions, run the Extension Development Host from the command palette:

In the Extension Development Host window, press {F1} to open the command palette, then type 'run a macro'.

Select the macro you want to debug from the list.

The program will stop at the breakpoint, allowing you to debug.
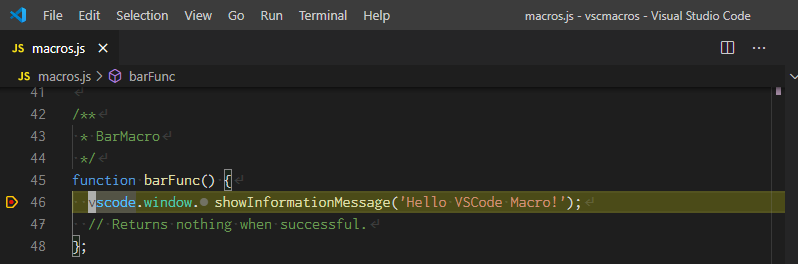
Macro examples
You can find examples of VSCode macros on GitHub Gist:
https://gist.github.com/exceedsystem
| |