Forge UI Snippets
This extension for Visual Studio Code adds snippets for Forge UI. Supports .jsx file extension.
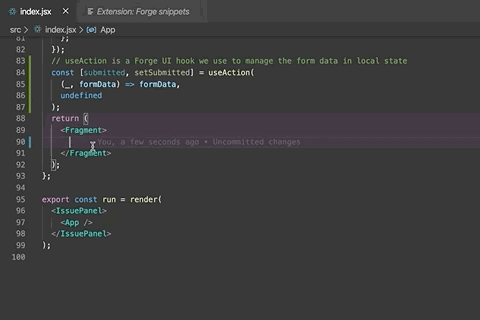
Table of Contents
Usage
Type part of a snippet, press enter, and the snippet unfolds.
Alternatively, press Ctrl+Space to activate snippets from within the editor.
Not supported components
Currently, the following components shortcuts are not available. Most of the time, following code components code, is generated while you create the specific type of Forge app.
- ContentAction
- ContextMenu
- InlineDialog
- IssueGlance
- IssuePanel
- Macro
Forge UI hooks
Type part of a snippet, press enter, and the snippet unfolds.
Shortcut Identifier |
Hook |
Code |
fustate→ |
useState |
useState |
fuaction→ |
useAction |
useState |
Installation
- Install Visual Studio Code 1.45.1 or higher
- Launch VS Code
- From the command palette Ctrl-Shift-P (Windows, Linux) or Cmd-Shift-P (macOS)
- Select Install Extension
- Choose the extension Forge Snippets
- Reload VS Code
Shortcut: fbtn→
Button component
<Button
text="button text"
onClick={() => console.log('perform button click action')}
/>
Shortcut: fbtns→
ButtonSet component
<ButtonSet>
<Button
text="button text 1"
onClick={() => console.log('perform button 1 click action')}
/>
<Button
ext="button text 2"
onClick={() => console.log('perform button 2 click action')}
/>
</ButtonSet>
TextCode
Shortcut: ftxt→
Text component
<Text>sample text</Text>
TextStrongCode
Shortcut: ftxts→
Text component
<Text>**It's a strong text**</Text>
TextEmphasisCode
Shortcut: ftxte→
Text component
<Text>*It's a emphasis text*</Text>
TextStrikeCode
Shortcut: ftxtstri→
Text component
<Text>~~strike~~</Text>
LinkCode
Shortcut: flink→
Text component
<Text>[It's a link to Forge](https://www.atlassian.com/forge)</Text>
Code
Shortcut: fcode→
Text component
<Text>{`<Text>sample text</Text>`}</Text>
UnorderedListCode
Shortcut: flistu→
Text component
<Text> - Item 1 </Text>
<Text> - Item 2 </Text>
<Text> - Item 3 </Text>
<Text> - Item 4 </Text>
OrderedListCode
Shortcut: flisto→
Text component
<Text> 1. Item 1 </Text>
<Text> 2. Item 2 </Text>
<Text> 3. Item 3 </Text>
<Text> 4. Item 4 </Text>
StatusLozengeCode
Shortcut: fstatus→
StatusLozenge component
<Text>
You have 4 tickets:{' '}
<StatusLozenge text="In progress" appearance="inprogress" />
</Text>
DateLozengeCode
Shortcut: fdate→
DateLozenge component
<Text>
<DateLozenge value={new Date('07-29-1988').getTime()} />
</Text>
FragmentCode
Shortcut: ffrgmnt→
Fragment component
<Fragment>
<Text content="Hello world!" />
<Button text="Do something" onClick={onClick} />
</Fragment>
ImageCode
Shortcut: fimg→
Image component
<Image
src="https://media.giphy.com/media/jUwpNzg9IcyrK/source.gif"
alt="homer"
/>
ModalDialogCode
Shortcut: fmodd→
ModalDialog component
<Button text="Show modal" onClick={() => setOpen(true)} />
{isOpen && (
<ModalDialog header=\"My modal dialog\" onClose={() => setOpen(false)}>
<Text>Hello there!</Text>
</ModalDialog>"
)}
TableCode
Shortcut: ftabl→
Table component
<Table>
<Head>
<Cell>
<Text content="Header Column 1=" />
</Cell>
<Cell>
<Text content="Header Column 2=" />
</Cell>
</Head>
<Row>
<Cell>
<Text content="Row 1 Column 1=" />
</Cell>
<Cell>
<Text content="Row 1 Column 2=" />
</Cell>
</Row>
</Table>
Shortcut: fform→
Form component
<Form onSubmit={setSubmitted}>
<TextField name="username" label="Username" />
<CheckboxGroup name="products" legend="Products">
<Checkbox defaultChecked value="jira" label="Jira" />
<Checkbox value="confluence" label="Confluence" />
</CheckboxGroup>
</Form>
FormTextFieldCode
Shortcut: fformtf→
Form TextField component
<TextField label="Name" name="name" />
FormTextAreaCode
Shortcut: fformta→
Form TextArea component
<TextArea label="Message" name="message" />
Shortcut: fforms→
Form Select component
<Select label="With a defaultSelected" name="milestone">
<Option defaultSelected label="Milestone 1" value="one" />
<Option label="Milestone 2" value="two" />
<Option label="Milestone 3" value="three" />
</Select>
Shortcut: fformrg→
Form RadioGroup component
<RadioGroup name="flavor" label="Pick a flavor">
<Radio defaultChecked label="Strawberry" value="strawberry" />
<Radio label="Cinnamon" value="cinnamon" />
<Radio label="Chocolate" value="chocolate" />
</RadioGroup>
Shortcut: fformdp→
Form DatePicker component
<DatePicker name="date" label="Appointment Date" />
Shortcut: fformcg→
Form CheckboxGroup component
<CheckboxGroup legend="Products" name="products">
<Checkbox value="jira" label="Jira" />
<Checkbox value="confluence" label="Confluence" />
</CheckboxGroup>
Shortcut: fformup→
Form UserPicker component
<UserPicker label="User" name="user" />
Shortcut: fconfigform→
ConfigForm component
const Config = () => {
return (
<ConfigForm>
<TextField name="name" label="Pet name" />
<TextField name="age" label="Pet age" />
</ConfigForm>
);
};
AvatarCode
Shortcut: favatar→
Avatar component
<Avatar accountId="5a1234bc8d12345e3f1g11hi" />
AvatarStackCode
Shortcut: favatars→
AvatarStack component
<AvatarStack>
<Avatar accountId="5a1234bc8d12345e3f1g11hi" />
<Avatar accountId="2a98a42dbc7ab42e12ee360d" />
<Avatar accountId="5d8732lq8jg85a0e3f1g90as" />
<Avatar accountId="2h98a10dbl5ab93e62hja23z" />
<Avatar accountId="7b20f0bc2d05325e3f1g43ty" />
<Avatar accountId="2p72s42dbc7ab42e90gf252d" />
<Avatar accountId="2l01x78mf4pqw42e84fg40ad" />
</AvatarStack>
If you have any suggestions or feedabck please contact @anilbms75.
useStateCode
Shortcut: fustate→
useState hook
const [count, setCount] = useState(0);
useActionCode
Shortcut: fuaction→
useAction hook
const [count, setCount] = useAction(
(currentCount, step) => currentCount + step,
0
);